Analog Sensor
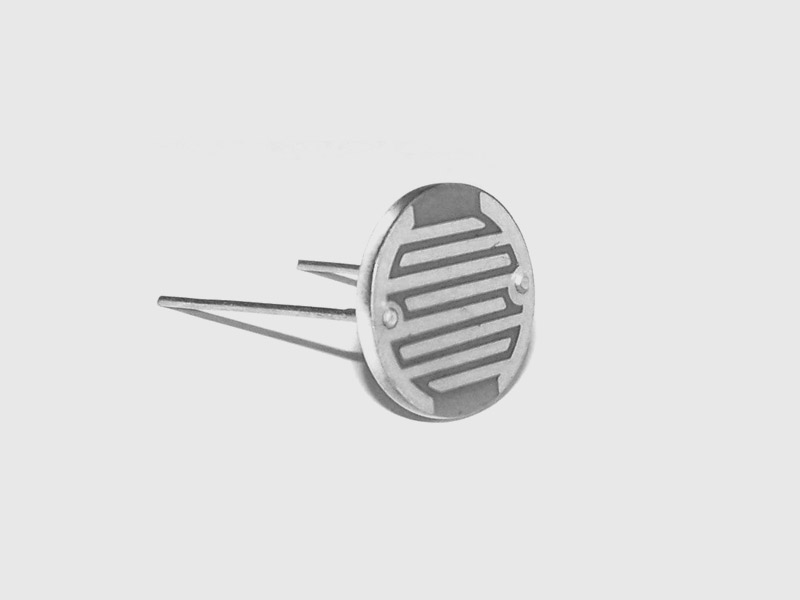
Makes it possible to interact with analog inputs on your device and obtain values that represent the analog input send by the sensors. The value received from an analog input read from the sensor goes from 0 to 1027.
For more information click here.
How To Connect
Cylon.robot({ connections: { arduino: { adaptor: 'firmata', port: '/dev/ttyACM0' } }, devices: { sensor: { driver: 'analog-sensor', pin: 0, lowerLimit: 100, upperLimit: 900 } } });
How To Use
This example detects lower and upper limits on a photoresistor sensor.
var Cylon = require('cylon'); Cylon.robot({ connections: { arduino: { adaptor: 'firmata', port: '/dev/ttyACM0' } }, devices: { sensor: { driver: 'analog-sensor', pin: 0, lowerLimit: 100, upperLimit: 900 } }, work: function(my) { var analogValue = 0; every((1).second(), function() { analogValue = my.sensor.analogRead(); console.log('Analog value => ', analogValue); }); my.sensor.on('lowerLimit', function(val) { console.log("Lower limit reached!"); console.log('Analog value => ', val); }); my.sensor.on('upperLimit', function(val) { console.log("Upper limit reached!"); console.log('Analog value => ', val); }); } }).start();
Commands
analogRead
Gets the current value from the Analog Sensor
Params
-
[callback] (
Function
) invoked witherr, value
as args
Returns
- (
Number
) the current sensor value
Events
analogRead
Emitted when the Analog Sensor has fetched a new value
upperLimit
Emitted when the Analog Sensor reads a value above the specified upper limit
lowerLimit
Emitted when the Analog Sensor reads a value below the specified lower limit
Circuit
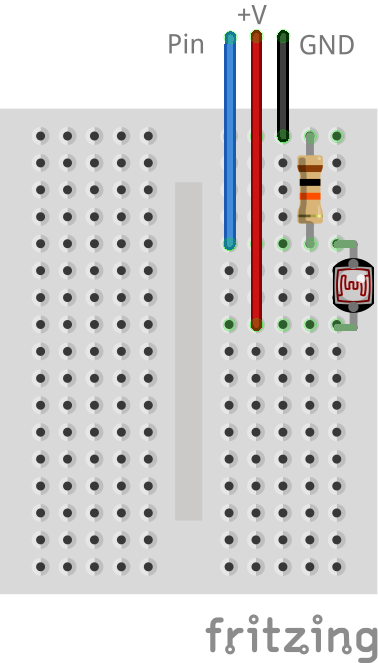