Installation
-
First, install Node.js
If you are on Linux, follow these instructions
If you already have Node.js installed, you can skip to the next step.
-
Next up, install the Cylon.js Command Line Interface (CLI) globally, so you can make use of the CLI commands it provides. You might need to use the "sudo" command if you are on OSX or Linux.
$ npm install -g cylon-cli
You might need to use the "sudo" command if you are on OSX or Linux.
$ sudo npm install -g cylon-cli
Once that's finished, you should be able to check if it's working by opening another terminal window and entering this command, which should give you a similar result:
$ cylon version 0.4.0
-
Now, create a local working directory that you want to use for the workshop:
$ mkdir thingscon $ cd thingscon
-
Install Cylon.js in the local working directory that you just created:
$ npm install cylon cylon-gpio cylon-i2c cylon-firmata cylon-sphero cylon-leapmotion
This command will install Cylon.js and all of the plugins you will need for the workshop.
-
To connect your Arduino to your computer, you will need to install the drivers which are part of the Arduino IDE software.
-
Finally, install the Leap Motion software. You do not need to signup for their "app store", you only need to install the drivers.
Arduino Blink
You can now test out your installation. Plug in your Arduino, and run the following code on your local computer. Cylon.js will communicate from your computer to the Arduino, and will make the built-in LED on your Arduino turn on/off every second:
JavaScript
//blink.js var Cylon = require('cylon'); Cylon.robot({ // change the port below to match whatever your Arduino is actually plugged into connections: { arduino: { adaptor: 'firmata', port: '/dev/ttyACM0' } }, devices: { led: { driver: 'led', pin: 13 } }, work: function(my) { every((1).seconds(), function() {my.led.toggle()}); } }); Cylon.start();
Please note that you might need to change the value for port.
On OSX or Linux, use
cylon scan serial
to find your connection info and serial port address:
$ cylon scan serial
On Windows, follow the following procedure:
- Go to the "Start" menu
- Right click on "Computer"
- Click on "Properties"
- Open "Device Manager" on the "Properties" tab
- Expand the tree controller listed in the "Device Manager" labeled "Ports (COM & LPT)"
- Note the port listed next to the "Arduino" entry. For example, "Arduino Uno (COM4)"
Makey Button
You will need to use your "Makey Button" kit to wire up your wearable controller. Here is an image of what the breadboard will end up looking like:
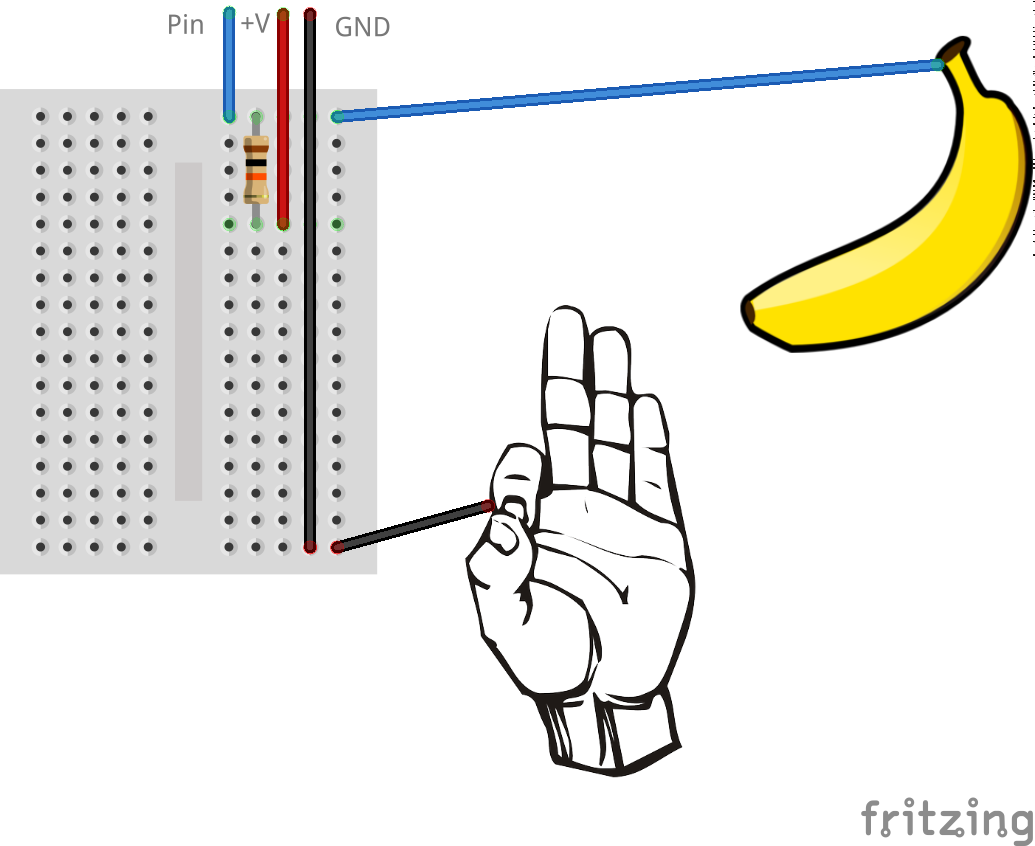
JavaScript
//makey.js var Cylon = require('cylon'); Cylon.robot({ connections: { arduino: { adaptor: 'firmata', port: '/dev/ttyACM0' } }, devices: { led: { driver: 'led', pin: 13 }, makey: { driver: 'makey-button', pin: 2 } }, work: function(my) { my.makey.on('push', function() { my.led.toggle(); }); } }); Cylon.start();
Connect To Sphero
OSX
In order to allow Cylon.js running on your Mac to access the Sphero, go to "Bluetooth > Open Bluetooth Preferences > Sharing Setup" and make sure that "Bluetooth Sharing" is checked.
Ubuntu
Connecting to the Sphero from Ubuntu or any other Linux-based OS can be done entirely from the command line using CylonJS CLI commands. Here are the steps.
Find the address of the Sphero, by using:
$ cylon bluetooth scan
Pair to Sphero using this command (substituting the actual address of your Sphero):
$ cylon bluetooth pair <address>
Connect to the Sphero using this command (substituting the actual address of your Sphero):
$ cylon bluetooth connect <address>Connect to your Sphero using Bluetooth. Here are instructions on how to connect
Sphero Color
JavaScript
//sphero_color.js var Cylon = require('cylon'); Cylon.robot({ connections: { sphero: { adaptor: 'sphero', port: '/dev/rfcomm0' } }, devices: { sphero: { driver: 'sphero' } }, work: function(me) { every((1).second(), function() { me.sphero.setRGB(Math.floor(Math.random() * 100000)); }); } }); Cylon.start();
Sphero Makey Button
JavaScript
//sphero_makey_button.js var Cylon = require('cylon'); var time = 0; var calibration = false; Cylon.robot({ connections: { arduino: { adaptor: 'firmata', port: '/dev/ttyACM0' }, sphero: { adaptor: 'sphero', port: '/dev/rfcomm0' } }, devices: { sphero: { driver: 'sphero', connection: 'sphero' }, forward: { driver: 'makey-button', connection: 'arduino', pin: 2 }, back: { driver: 'makey-button', connection: 'arduino', pin: 3 }, left: { driver: 'makey-button', connection: 'arduino', pin: 4 }, right: { driver: 'makey-button', connection: 'arduino', pin: 5 } }, checkCalibration: (function(_this) { return function(me) { if (calibration === true) { me.sphero.finishCalibration(); calibration = false; } }; })(this), work: function(my) { my.checkCalibration(my); my.sphero.setBackLED(255); my.forward.on('push', function() { console.log("forward"); my.checkCalibration(my); my.sphero.roll(90, 0); }); my.forward.on('release', function() { my.sphero.stop(); }); my.back.on('push', function() { console.log("back"); my.checkCalibration(my); my.sphero.roll(90, 180); }); my.back.on('release', function() { my.sphero.stop(); }); my.right.on('push', function() { console.log("right"); var t = Date.now(); if ((t - time) < 400 ) { console.log("Calibrating"); my.sphero.startCalibration(); calibration = true; } else { my.checkCalibration(my); my.sphero.roll(90, 90); } time = t; }); my.right.on('release', function() { my.sphero.stop(); }); my.left.on('push', function() { console.log("left"); my.checkCalibration(my); my.sphero.roll(90, 270); }); my.left.on('release', function() { my.sphero.stop(); }); } }); Cylon.start();
Leap Motion
JavaScript
//leap.js var Cylon = require('cylon'); Cylon.robot({ connections: { leapmotion: { adaptor: 'leapmotion' } }, devices: { leapmotion: { driver: 'leapmotion' } }, work: function(my) { my.leapmotion.on('connect', function() { console.log("Connected"); }); my.leapmotion.on('start', function() { console.log("Started"); }); my.leapmotion.on('frame', function(frame) { console.log(frame.toString()); }); my.leapmotion.on('hand', function(hand) { console.log(hand.toString()); }); my.leapmotion.on('pointable', function(pointable) { console.log(pointable.toString()); }); my.leapmotion.on('gesture', function(gesture) { console.log(gesture.toString()); }); } }); Cylon.start();
Leap Motion Sphero
JavaScript
//leap_sphero.js var Cylon = require('cylon'); Cylon.robot({ connections: { leapmotion: { adaptor: 'leapmotion' }, sphero: { adaptor: 'sphero', port: '/dev/rfcomm0' } }, devices: { leapmotion: { driver: 'leapmotion', connection: 'leapmotion' }, sphero: { driver: 'sphero', connection: 'sphero' } }, work: function(my) { my.leapmotion.on('hand', function(hand) { var r = hand.palmY.fromScale(100, 600).toScale(0, 255) | 0; my.sphero.setRGB(r, 0, 0); }); } }) Cylon.start();