Bebop - Flight
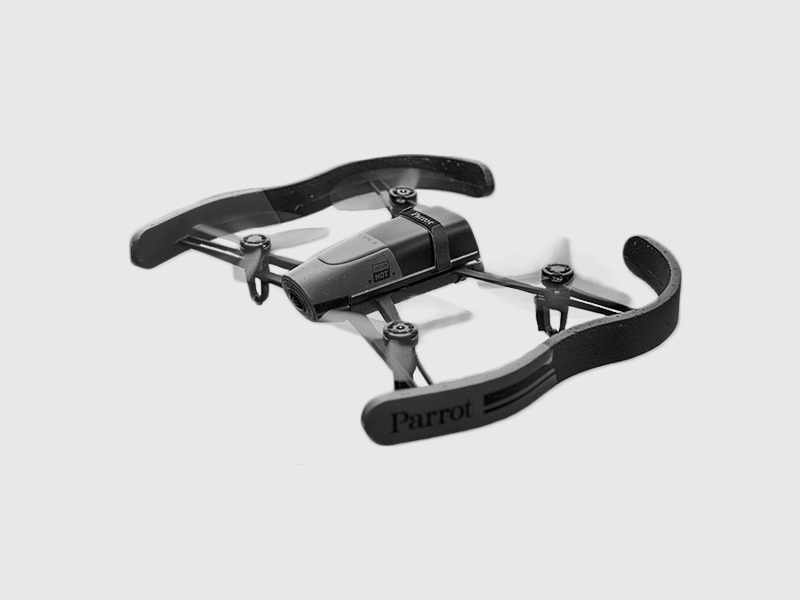
Allows user to send flight control commands to Bebop. Flight control commands are those used to tell the Bebop to take off, land, and or any number of other autonomous flight manuvers.
For more information click here.
How To Connect
var Cylon = require('cylon'); Cylon.robot({ connections: { bebop: { adaptor: 'bebop' } }, devices: { drone: { driver: 'bebop' } }, });
How To Use
This example controls a Bebop to take off, and then land.
var Cylon = require('cylon'); Cylon.robot({ connections: { bebop: { adaptor: 'bebop' } }, devices: { drone: { driver: 'bebop' } }, work: function(my) { my.drone.takeOff(); after((5).seconds(), my.drone.land); } }).start();
Commands
takeOff
Tells Bebop to start flying by setting the internal 'fly' state to true.
Params
-
callback (
Function
) function to invoke once hovering
Returns
- (
Boolean
)
land
Tells Bebop to land by setting the internal 'fly' state to false.
Params
-
callback (
Function
) function to invoke once landed
Returns
- (
Boolean
)
stop
Sets all drone movement commands to 0, making it effectively hover in place.
Returns
- (
null
)
up
Tells the drone to start gaining altitude
Params
-
speed (
Number
) value from 0 to 1
Returns
- (
Number
) set speed
down
Tells the drone to start losing altitude
Params
-
speed (
Number
) value from 0 to 1
Returns
- (
Number
) set speed
left
Tells the drone to bank left - controlling the roll using the camera as a reference point.
Params
-
speed (
Number
) value from 0 to 1
Returns
- (
Number
) set speed
right
Tells the drone to bank right - controlling the roll using the camera as a reference point.
Params
-
speed (
Number
) value from 0 to 1
Returns
- (
Number
) set speed
forward
Tells the drone to bank forward - controlling the pitch using the camera as a reference point.
Params
-
speed (
Number
) value from 0 to 1
Returns
- (
Number
) set speed
backward
Tells the drone to bank backwards - controlling the pitch using the camera as a reference point.
Params
-
speed (
Number
) value from 0 to 1
Returns
- (
Number
) set speed
clockwise
Tells the drone to begin spinning clockwise
Params
-
speed (
Number
) value from 0 to 1
Returns
- (
Number
) set speed
counterClockwise
Tells the drone to begin spinning counterclockwise
Params
-
speed (
Number
) value from 0 to 1
Returns
- (
Number
) set speed
frontFlip
Tells the drone to frontFlip
Returns
- (
Number
) flip direction
backFlip
Tells the drone to backFlip
Returns
- (
Number
) flip direction
rightFlip
Tells drone to rightflip
Returns
- (
Number
) flip direction
leftFlip
Tells drone to leftflip
Returns
- (
Number
) flip direction
emergency
Tell the drone to drop like a stone.
Returns
- (
string
) buffer of emergency calls