Sphero
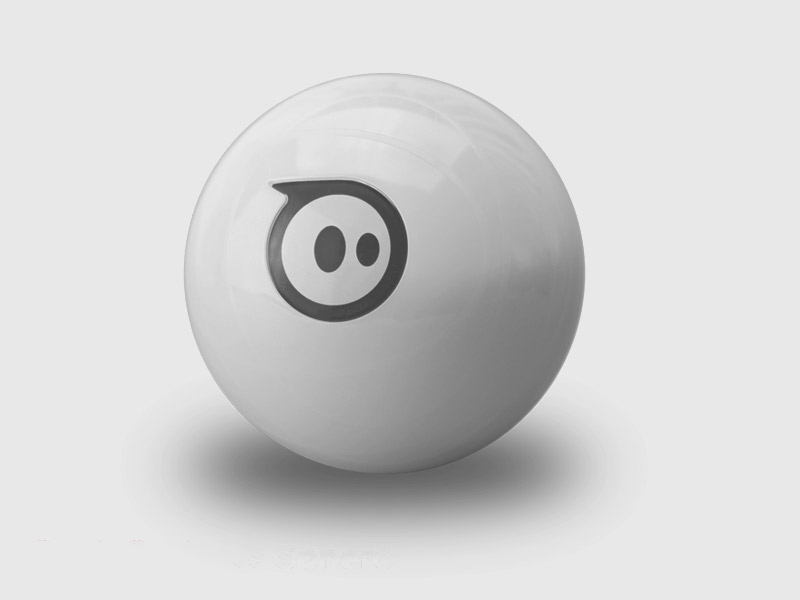
Allows user to interact with the Sphero robotic sphero using Cylon.js.
For more information click here.
How To Connect
That is pretty simple once the Sphero is ready (connected and listening), you just need three lines of code and you are set,
Cylon.js and the Sphero driver take care of the rest.
Cylon.robot({ connections: { sphero: { adaptor: 'sphero', port: '/dev/rfcomm0' } }, devices: { sphero: { driver: 'sphero' } }, });
How To Use
This example rolls the sphero in a random direction and change direction every second.
var Cylon = require('cylon'); Cylon.robot({ connections: { sphero: { adaptor: 'sphero', port: '/dev/rfcomm0' } }, devices: { sphero: { driver: 'sphero' } }, work: function(me) { every((1).second(), function() { me.sphero.roll 60, Math.floor(Math.random() * 360) }); } }).start();
Commands
abortMacro
The Abort Macro command aborts any executing macro, and returns both it's ID code and the command number currently in progress.
An exception is a System Macro executing with the UNKILLABLE flag set.
A returned ID code of 0x00 indicates that no macro was running, an ID code of 0xFFFF as the CmdNum indicates the macro was unkillable.
Params
-
callback (
Function
) function to be triggered with response
Returns
- (
undefined
)
abortOrbBasicProgram
The Abort orbBasic Program command aborts execution of any currently running orbBasic program.
Params
-
callback (
Function
) function to be triggered with response
Returns
- (
undefined
)
appendMacroChunk
The Append Macro Chunk project stores the attached macro definition into the temporary RAM buffer for later execution.
It's similar to the Save Temporary Macro command, but allows building up longer temporary macros.
Any existing Macro ID can be sent through this command, and executed through the Run Macro call using ID 0xFF.
If this command is sent while a Temporary or Stream Macro is executing it will be terminated so that its storage space can be overwritten. As with all macros, the longest chunk that can be sent is 254 bytes.
You must follow this with a Run Macro command (ID 0xFF) to actually get it to go and it is best to prefix this command with an Abort call to make certain the larger buffer is completely initialized.
Params
-
chunk (
Array
) of bytes to append for macro execution -
callback (
Function
) function to be triggered with response
Returns
- (
undefined
)
appendOrbBasicFragment
The Append orbBasic Fragment command appends a patch of orbBasic code to existing ones in the specified storage area (0x00 for RAM, 0x01 for persistent).
Complete lines are not required. A line begins with a decimal line number
followed by a space and is terminated with a
See the orbBasic Interpreter document for complete information.
Possible error responses would be ORBOTIX_RSP_CODE_EPARAM if an illegal storage area is specified or ORBOTIX_RSP_CODE_EEXEC if the specified storage area is full.
Params
-
area (
Number
) which area to append the fragment to -
code (
String
) orbBasic code to append -
callback (
Function
) function to be triggered with response
Returns
- (
undefined
)
assignTime
The Assign Time command sets a specific value to Sphero's internal 32-bit relative time counter.
Params
-
time (
Number
) the new value to set -
callback (
Function
) function to be triggered when done writing
Returns
- (
undefined
)
boost
The Boost command executes Sphero's boost macro.
It takes a 1-byte parameter, 0x01 to start boosting, or 0x00 to stop.
Params
-
boost (
Number
) whether or not to boost (1 - yes, 0 - no) -
callback (
Function
) function to be triggered after writing
Returns
- (
undefined
)
clearCounters
The Clear Counters command is a developer-only command to clear the various system counters created by the L2 diagnostics.
It is denied when the Sphero is in Normal mode.
Params
-
callback (
Function
) function to be triggered when done writing
Returns
- (
undefined
)
color
The Color command wraps Sphero's built-in setRgb command, allowing for a greater range of possible inputs.
Params
-
color ( Number
,String
Object
) what color to change Sphero to -
callback (
Function
) function to be triggered with response
Returns
- (
undefined
)
commitToFlash
The Commit To Flash command copies the current orbBasic RAM program to persistent flash storage.
It will fail if a program is currently executing out of flash.
Params
-
callback (
Function
) function to be triggered with response
Returns
- (
undefined
)
configureCollisions
The Configure Collisions command configures Sphero's collision detection with the provided parameters.
These include:
- meth - which detection method to use. Supported methods are 0x01, 0x02, and 0x03 (see the collision detection document for details). 0x00 disables this service.
- xt, yt - 8-bit settable threshold for the X (left, right) and y (front, back) axes of Sphero. 0x00 disables the contribution of that axis.
-
xs, ys - 8-bit settable speed value for X/Y axes. This setting is
ranged by the speed, than added to
xt
andyt
to generate the final threshold value. - dead - an 8-bit post-collision dead time to prevent re-triggering. Specified in 10ms increments.
Params
-
opts (
Object
) object containing collision configuration opts -
cb (
Function
) function to be triggered after writing
Returns
- (
undefined
)
configureLocator
The Configure Locator command configures Sphero's streaming location data service.
The following options must be provided:
- flags - bit 0 determines whether calibrate commands auto-correct the yaw tare value. When false, positive Y axis coincides with heading 0. Other bits are reserved.
- x, y - the current (x/y) coordinates of Sphero on the ground plane in centimeters
- yawTare - controls how the x,y-plane is aligned with Sphero's heading coordinate system. When zero, yaw = 0 corresponds to facing down the y-axis in the positive direction. Possible values are 0-359 inclusive.
Params
-
opts (
Object
) object containing locator service configuration -
callback (
Function
) function to be triggered after writing
Returns
- (
undefined
)
controlUartTx
The Control UART Tx command enables or disables the CPU's UART transmit line so another client can configure the Bluetooth module.
Params
-
callback (
Function
) function to be triggered after write
Returns
- (
undefined
)
detectCollisions
The Detect Collisions command sets up Sphero's collision detection system, and automatically parses asynchronous packets to re-emit collision events to 'collision' event listeners.
Params
-
callback (
Function
) (err, data) to be triggered with response
Returns
- (
undefined
)
enableSsbAsyncMsg
The Enable SSB Async Messages command turns on/off soul block related asynchronous messages.
These include shield collision/regrowth messages, boost use/regrowth messages, XP growth, and level-up messages.
This feature defaults to off.
Params
-
flag (
Number
) whether or not to enable async messages -
callback (
Function
) function to be triggered after write
Returns
- (
undefined
)
eraseOrbBasicStorage
The Erase orbBasic Storage command erases any existing program in the specified storage area.
Specify 0x00 for the temporary RAM buffer, or 0x01 for the persistent storage area.
Params
-
area (
Number
) which area to erase -
callback (
Function
) function to be triggered with response
Returns
- (
undefined
)
executeOrbBasicProgram
The Execute orbBasic Program command attempts to run a program in the specified storage area, beginning at the specified line number.
This command will fail if there is already an orbBasic program running.
Params
-
area (
Number
) which area to run from -
slMSB (
Number
) start line -
slLSB (
Number
) start line -
callback (
Function
) function to be triggered with response
Returns
- (
undefined
)
finishCalibration
The Finish Calibration command ends Sphero's calibration mode, by setting the new heading as current, turning off the back LED, and re-enabling stabilization.
Params
-
callback (
Function
) function to be triggered with response
Returns
- (
undefined
)
getAutoReconnect
The Get Auto Reconnect command returns the Bluetooth auto reconnect values as defined above in the Set Auto Reconnect command.
Params
-
callback (
Function
) function to be triggered with reconnect data
Returns
- (
undefined
)
getBluetoothInfo
Triggers the callback with a structure containing
- Sphero's ASCII name
- Sphero's Bluetooth address (ASCII)
- Sphero's ID colors
Params
-
callback (
Function
) function to be triggered with Bluetooth info
Returns
- (
undefined
)
getChassisId
The Get Chassis ID command returns the 16-bit chassis ID Sphero was assigned at the factory.
Params
-
callback (
Function
) function to be triggered with a response
Returns
- (
undefined
)
getColor
Passes the color of the sphero Rgb LED to the callback (err, data)
Params
-
callback (
Function
) function to be triggered with response
Returns
- (
undefined
)
getConfigBlock
The Get Configuration Block command retrieves one of Sphero's configuration blocks.
The response is a simple one; an error code of 0x08 is returned when the resources are currently unavailable to send the requested block back. The actual configuration block data returns in an asynchronous message of type 0x04 due to its length (if there is no error).
ID = 0x00
requests the factory configuration block
ID = 0x01
requests the user configuration block, which is updated with
current values first
Params
-
id (
Number
) which configuration block to fetch -
callback (
Function
) function to be triggered after writing
Returns
- (
undefined
)
getDeviceMode
The Get Device Mode command gets the current device mode of Sphero.
Possible values:
- 0x00: Normal mode
- 0x01: User Hack mode.
Params
-
callback (
Function
) function to be called with response
Returns
- (
undefined
)
getMacroStatus
The Get Macro Status command returns the ID code and command number of the currently executing macro.
If no macro is running, the 0x00 is returned for the ID code, and the command number is left over from the previous macro.
Params
-
callback (
Function
) function to be triggered with response
Returns
- (
undefined
)
getPermOptionFlags
The Get Permanent Option Flags command returns Sphero's permanent option flags, as a bit field.
Here's possible bit fields, and their descriptions:
-
0
: Set to prevent Sphero from immediately going to sleep when placed in the charger and connected over Bluetooth. -
1
: Set to enable Vector Drive, that is, when Sphero is stopped and a new roll command is issued it achieves the heading before moving along it. -
2
: Set to disable self-leveling when Sphero is inserted into the charger. -
3
: Set to force the tail LED always on. -
4
: Set to enable motion timeouts (see DID 02h, CID 34h) -
5
: Set to enable retail Demo Mode (when placed in the charger, ball runs a slow rainbow macro for 60 minutes and then goes to sleep). -
6
: Set double tap awake sensitivity to Light -
7
: Set double tap awake sensitivity to Heavy -
8
: Enable gyro max async message (NOT SUPPORTED IN VERSION 1.47) -
6-31
: Unassigned
Params
-
callback (
Function
) function triggered with option flags data
Returns
- (
undefined
)
getPowerState
The Get Power State command returns Sphero's current power state, and some additional parameters:
- RecVer: record version code (following is for 0x01)
- Power State: high-level state of the power system
- BattVoltage: current battery voltage, scaled in 100ths of a volt (e.g. 0x02EF would be 7.51 volts)
- NumCharges: Number of battery recharges in the life of this Sphero
- TimeSinceChg: Seconds awake since last recharge
Possible power states:
- 0x01 - Battery Charging
- 0x02 - Battery OK
- 0x03 - Battery Low
- 0x04 - Battery Critical
Params
-
callback (
Function
) function to be triggered with power state data
Returns
- (
undefined
)
getRgbLed
The Get RGB LED command fetches the current "user LED color" value, stored in Sphero's configuration.
This value may or may not be what's currently displayed by Sphero's LEDs.
Params
-
callback (
Function
) function to be triggered after writing
Returns
- (
undefined
)
getSsb
The Get SSB command retrieves Sphero's Soul Block.
The response is simple, and then the actual block of soulular data returns in an asynchronous message of type 0x0D, due to it's 0x440 byte length
Params
-
callback (
Function
) function to be called with response
Returns
- (
undefined
)
getTempOptionFlags
The Get Temporary Option Flags command returns Sphero's temporary option flags, as a bit field:
-
0
: Enable Stop On Disconnect behavior -
1-31
: Unassigned
Params
-
callback (
Function
) function triggered with option flags data
Returns
- (
undefined
)
getVoltageTripPoints
The Get Voltage Trip Points command returns the trip points Sphero uses to determine Low battery and Critical battery.
The values are expressed in 100ths of a volt, so defaults of 7V and 6.5V respectively are returned as 700 and 650.
Params
-
callback (
Function
) function to be triggered with trip point data
Returns
- (
undefined
)
jumpToBootloader
The Jump To Bootloader command requests a jump into the Bootloader to prepare for a firmware download.
All commands after this one must comply with the Bootloader Protocol Specification.
Params
-
callback (
Function
) function to be triggered when done writing
Returns
- (
undefined
)
ping
The Ping command verifies the Sphero is awake and receiving commands.
Params
-
callback (
Function
) triggered when Sphero has been pinged
Returns
- (
undefined
)
pollPacketTimes
The Poll Packet Times command helps users profile the transmission and processing latencies in Sphero.
For more details, see the Sphero API documentation.
Params
-
time (
Number
) a timestamp to use for profiling -
callback (
Function
) function to be triggered when done writing
Returns
- (
undefined
)
randomColor
The Random Color command sets Sphero to a randomly-generated color.
Params
-
callback (
Function
) (err, data) to be triggered with response
Returns
- (
undefined
)
reInitMacroExec
The Reinit Macro Executive command terminates any running macro, and reinitializes the macro system.
The table of any persistent user macros is cleared.
Params
-
callback (
Function
) function to be triggered with response
Returns
- (
undefined
)
readLocator
The Read Locator command gets Sphero's current position (X,Y), component velocities, and speed-over-ground (SOG).
The position is a signed value in centimeters, the component velocities are signed cm/sec, and the SOG is unsigned cm/sec.
Params
-
callback (
Function
) function to be triggered with data
Returns
- (
undefined
)
roll
The Roll command tells Sphero to roll along the provided vector.
Both a speed and heading are required, the latter is considered relative to the last calibrated direction.
Permissible heading values are 0 to 359 inclusive.
Params
-
speed (
Number
) what speed Sphero should roll at -
heading (
Number
) what heading Sphero should roll towards (0-359) -
[state] (
Number
) optional state parameter -
callback (
Function
) function to be triggered after writing
Returns
- (
undefined
)
runL1Diags
The Perform Level 1 Diagnostics command is a developer-level command to help diagnose aberrant behaviour in Sphero.
Most process flags, system counters, and system states are decoded to human-readable ASCII.
For more details, see the Sphero API documentation.
Params
-
callback (
Function
) function to be triggered with diagnostic data
Returns
- (
undefined
)
runL2Diags
The Perform Level 2 Diagnostics command is a developer-level command to help diagnose aberrant behaviour in Sphero.
It's much less informative than the Level 1 command, but is in binary format and easier to parse.
For more details, see the Sphero API documentation.
Params
-
callback (
Function
) function to be triggered with diagnostic data
Returns
- (
undefined
)
runMacro
The Run Macro command attempts to execute the specified macro.
Macro IDs are split into groups:
0-31 are System Macros. They are compiled into the Main Application, and cannot be deleted. They are always available to run.
32-253 are User Macros. They are downloaded and persistently stored, and can be deleted in total.
255 is the Temporary Macro, a special user macro as it is held in RAM for execution.
254 is also a special user macro, called the Stream Macro that doesn't require this call to begin execution.
This command will fail if there is a currently executing macro, or the specified ID code can't be found.
Params
-
id (
Number
) 8-bit Macro ID to run -
callback (
Function
) function to be triggered with response
Returns
- (
undefined
)
saveMacro
Save macro
The Save Macro command stores the attached macro definition into the persistent store for later execution. This command can be sent even if other macros are executing.
You will receive a failure response if you attempt to send an ID number in the System Macro range, 255 for the Temp Macro and ID of an existing user macro in the storage block.
As with all macros, the longest definition that can be sent is 254 bytes.
Params
-
macro (
Array
) array of bytes with the data to be written -
callback (
Function
) function to be triggered with response
Returns
- (
undefined
)
saveTempMacro
The Save Temporary Macro stores the attached macro definition into the temporary RAM buffer for later execution.
If this command is sent while a Temporary or Stream Macro is executing it will be terminated so that its storage space can be overwritten. As with all macros, the longest definition that can be sent is 254 bytes.
Params
-
macro (
Array
) array of bytes with the data to be written -
callback (
Function
) function to be triggered with response
Returns
- (
undefined
)
selfLevel
The Self Level command controls Sphero's self-level routine.
This routine attempts to achieve a horizontal orientation where pitch/roll angles are less than the provided Angle Limit.
After both limits are satisfied, option bits control sleep, final angle(heading), and control system on/off.
An asynchronous message is returned when the self level routine completes.
For more detail on opts param, see the Sphero API documentation.
opts: - angleLimit: 0 for defaul, 1 - 90 to set. - timeout: 0 for default, 1 - 255 to set. - trueTime: 0 for default, 1 - 255 to set. - options: bitmask 4bit e.g. 0xF; };
Params
-
opts (
Object
) self-level routine options -
callback (
Function
) function to be triggered after writing
Returns
- (
undefined
)
setAccelRange
The Set Accelerometer Range command tells Sphero what accelerometer range to use.
By default, Sphero's solid-state accelerometer is set for a range of ±8Gs. You may wish to change this, perhaps to resolve finer accelerations.
This command takes an index for the supported range, as explained below:
-
0
: ±2Gs -
1
: ±4Gs -
2
: ±8Gs (default) -
3
: ±16Gs
Params
-
idx (
Number
) what accelerometer range to use -
callback (
Function
) function to be triggered after writing
Returns
- (
undefined
)
setAutoReconnect
The Set Auto Reconnect command tells Sphero's BT module whether or not it should automatically reconnect to the previously-connected Apple mobile device.
Params
-
flag (
Number
) whether or not to reconnect (0 - no, 1 - yes) -
time (
Number
) how many seconds after start to enable auto reconnect -
callback (
Function
) function to be triggered after write
Returns
- (
undefined
)
setBackLed
The Set Back LED command allows brightness adjustment of Sphero's tail light.
This value does not persist across power cycles.
Params
-
brightness (
Number
) brightness to set to Sphero's tail light -
callback (
Function
) function to be triggered after writing
Returns
- (
undefined
)
setChassisId
The Set Chassis ID command assigns Sphero's chassis ID, a 16-bit value.
This command only works if you're at the factory.
Params
-
chassisId (
Number
) new chassis ID -
callback (
Function
) function to be triggered after writing
Returns
- (
undefined
)
setConfigBlock
The Set Config Block command accepts an exact copy of the configuration block, and loads it into the RAM copy of the configuration block.
The RAM copy is then saved to flash.
The configuration block can be obtained by using the Get Configuration Block command.
Params
-
block (
Array
) - An array of bytes with the data to be written -
callback (
Function
) - To be triggered when done
Returns
- (
undefined
)
setDataStreaming
The Set Data Streaming command configures Sphero's built-in support for asynchronously streaming certain system and sensor data.
This command selects the internal sampling frequency, packet size, parameter mask, and (optionally) the total number of packets.
These options are provided as an object, with the following properties:
- n - divisor of the maximum sensor sampling rate
- m - number of sample frames emitted per packet
- mask1 - bitwise selector of data sources to stream
- pcnt - packet count 1-255 (or 0, for unlimited streaming)
- mask2 - bitwise selector of more data sources to stream (optional)
For more explanation of these options, please see the Sphero API documentation.
Params
-
opts (
Object
) object containing streaming data options -
callback (
Function
) function to be triggered after writing
Returns
- (
undefined
)
setDeviceMode
The Set Device Mode command assigns the operation mode of Sphero based on the supplied mode value.
- 0x00: Normal mode
- 0x01: User Hack mode. Enables ASCII shell commands, refer to the associated document for details.
Params
-
mode (
Number
) which mode to set Sphero to -
callback (
Function
) function to be called after writing
Returns
- (
undefined
)
setDeviceName
The Set Device Name command assigns Sphero an internal name. This value is then produced as part of the Get Bluetooth Info command.
Names are clipped at 48 characters to support UTF-8 sequences. Any extra characters will be discarded.
This field defaults to the Bluetooth advertising name of Sphero.
Params
-
name (
String
) what name to give to the Sphero -
callback (
Function
) function to be triggered when the name is set
Returns
- (
undefined
)
setHeading
The Set Heading command tells Sphero to adjust it's orientation, by commanding a new reference heading (in degrees).
If stabilization is enabled, Sphero will respond immediately to this.
Params
-
heading (
Number
) Sphero's new reference heading, in degrees (0-359) -
callback (
Function
) function to be triggered after writing
Returns
- (
undefined
)
setInactivityTimeout
The Set Inactivity Timeout command sets the timeout delay before Sphero goes to sleep automatically.
By default, the value is 600 seconds (10 minutes), but this command can alter it to any value of 60 seconds or greater.
Params
-
time (
Number
) new delay before sleeping -
callback (
Function
) function to be triggered when done writing
Returns
- (
undefined
)
setMacroParam
The Set Macro Parameter command allows system globals that influence certain macro commands to be selectively altered from outside of the macro system itself.
The values of Val1 and Val2 depend on the parameter index.
Possible indices:
- 00h Assign System Delay 1: Val1 = MSB, Val2 = LSB
- 01h Assign System Delay 2: Val1 = MSB, Val2 = LSB
- 02h Assign System Speed 1: Val1 = speed, Val2 = 0 (ignored)
- 03h Assign System Speed 2: Val1 = speed, Val2 = 0 (ignored)
- 04h Assign System Loops: Val1 = loop count, Val2 = 0 (ignored)
For more details, please refer to the Sphero Macro document.
Params
-
index (
Number
) what parameter index to use -
val1 (
Number
) value 1 to set -
val2 (
Number
) value 2 to set -
callback (
Function
) function to be triggered with response
Returns
- (
undefined
)
setMotionTimeout
The Set Motion Timeout command gives Sphero an ultimate timeout for the last motion command to keep Sphero from rolling away in the case of a crashed (or paused) application.
This defaults to 2000ms (2 seconds) upon wakeup.
Params
-
time (
Number
) timeout length in milliseconds -
callback (
Function
) function to be triggered when done writing
Returns
- (
undefined
)
setPermOptionFlags
The Set Permanent Option Flags command assigns Sphero's permanent option flags to the provided values, and writes them immediately to the config block.
See below for the bit definitions.
Params
-
flags (
Array
) permanent option flags -
callback (
Function
) function to be triggered when done writing
Returns
- (
undefined
)
setPowerNotification
The Set Power Notification command enables sphero to asynchronously notify the user of power state periodically (or immediately, when a change occurs)
Timed notifications are sent every 10 seconds, until they're disabled or Sphero is unpaired.
Params
-
flag (
Number
) whether or not to send notifications (0 - no, 1 - yes) -
callback (
Function
) function to be triggered when done writing
Returns
- (
undefined
)
setRawMotors
The Set Raw Motors command allows manual control over one or both of Sphero's motor output values.
Each motor (left and right requires a mode and a power value from 0-255.
This command will disable stabilization is both mode's aren't "ignore", so you'll need to re-enable it once you're done.
Possible modes:
-
0x00
: Off (motor is open circuit) -
0x01
: Forward -
0x02
: Reverse -
0x03
: Brake (motor is shorted) -
0x04
: Ignore (motor mode and power is left unchanged
Params
-
opts (
Object
) object with mode/power values (e.g. lmode, lpower) -
callback (
Function
) function to be triggered after writing
Returns
- (
undefined
)
setRgbLed
The Set RGB LED command sets the colors of Sphero's RGB LED.
An object containaing red
, green
, and blue
values must be provided.
If opts.flag
is set to 1 (default), the color is persisted across power
cycles.
Params
-
opts (
Object
) object containing RGB values for Sphero's LED -
callback (
Function
) function to be triggered after writing
Returns
- (
undefined
)
setRotationRate
The Set Rotation Rate command allows control of the rotation rate Sphero uses to meet new heading commands.
A lower value offers better control, but with a larger turning radius.
Higher values yield quick turns, but Sphero may lose control.
The provided value is in units of 0.784 degrees/sec.
Params
-
rotation (
Number
) new rotation rate (0-255) -
callback (
Function
) function to be triggered after writing
Returns
- (
undefined
)
setStabilization
The Set Stabilization command turns Sphero's internal stabilization on or off, depending on the flag provided.
Params
-
flag (
Number
) stabilization setting flag (0 - off, 1 - on) -
callback (
Function
) function to be triggered after writing
Returns
- (
undefined
)
setTempOptionFlags
The Set Temporary Option Flags command assigns Sphero's temporary option flags to the provided values. These do not persist across power cycles.
See below for the bit definitions.
Params
-
flags (
Array
) permanent option flags -
callback (
Function
) function to be triggered when done writing
Returns
- (
undefined
)
setVoltageTripPoints
The Set Voltage Trip Points command assigns the voltage trip points for Low and Critical battery voltages.
Values are specified in 100ths of a volt, and there are limitations on adjusting these from their defaults:
- vLow must be in the range 675-725
- vCrit must be in the range 625-675
There must be 0.25v of separation between the values.
Shifting these values too low can result in very little warning before Sphero forces itself to sleep, depending on the battery pack. Be careful.
Params
-
vLow (
Number
) new voltage trigger for Low battery -
vCrit (
Number
) new voltage trigger for Crit battery -
callback (
Function
) function to be triggered when done writing
Returns
- (
undefined
)
sleep
The Sleep command puts Sphero to sleep immediately.
Params
-
wakeup (
Number
) the number of seconds for Sphero to re-awaken after. 0x00 tells Sphero to sleep forever, 0xFFFF attemps to put Sphero into deep sleep. -
macro (
Number
) if non-zero, Sphero will attempt to run this macro ID when it wakes up -
orbBasic (
Number
) if non-zero, Sphero will attempt to run an orbBasic program from this line number -
callback (
Function
) function to be triggered when done writing
Returns
- (
undefined
)
startCalibration
The Start Calibration command sets up Sphero for manual heading calibration.
It does this by turning on the tail light (so you can tell where it's facing) and disabling stabilization (so you can adjust the heading).
When done, call #finishCalibration to set the new heading, and re-enable stabilization.
Params
-
callback (
Function
) (err, data) to be triggered with response
Returns
- (
undefined
)
stop
Stops sphero the optimal way by setting flag 'go' to 0 and speed to a very low value.
Params
-
callback (
Function
) triggered on complete
Returns
- (
undefined
)
stopOnDisconnect
The Stop On Disconnect command sends a flag to Sphero. This flag tells Sphero whether or not it should automatically stop when it detects that it's disconnected.
Params
-
[remove=false] (
Boolean
) whether or not to stop on disconnect -
callback (
Function
) triggered on complete
Returns
- (
undefined
)
streamAccelOne
Starts streaming of accelOne data
It uses sphero's data streaming command. User needs to listen
for the dataStreaming
or accelOne
event to get the data.
Params
-
[sps=5] (
Number
) samples per second -
[remove=false] (
Boolean
) forces velocity streaming to stop
Returns
- (
undefined
)
streamAccelerometer
Starts streaming of accelerometer data
It uses sphero's data streaming command. User needs to listen
for the dataStreaming
or accelerometer
event to get the data.
Params
-
[sps=5] (
Number
) samples per second -
[remove=false] (
Boolean
) forces velocity streaming to stop
Returns
- (
undefined
)
streamData
Generic Data Streaming setup, using Sphero's setDataStraming command.
Users need to listen for the dataStreaming
event, or a custom event, to
get the data.
Params
-
args (
Object
) event, masks, fields, and sps data
Returns
- (
undefined
)
streamGyroscope
Starts streaming of gyroscope data
It uses sphero's data streaming command. User needs to listen
for the dataStreaming
or gyroscope
event to get the data.
Params
-
[sps=5] (
Number
) samples per second -
[remove=false] (
Boolean
) forces velocity streaming to stop
Returns
- (
undefined
)
streamImuAngles
Starts streaming of IMU angles data
It uses sphero's data streaming command. User needs to listen
for the dataStreaming
or imuAngles
event to get the data.
Params
-
[sps=5] (
Number
) samples per second -
[remove=false] (
Boolean
) forces velocity streaming to stop
Returns
- (
undefined
)
streamMotorsBackEmf
Starts streaming of motor back EMF data
It uses sphero's data streaming command. User needs to listen
for the dataStreaming
or motorsBackEmf
event to get the data.
Params
-
[sps=5] (
Number
) samples per second -
[remove=false] (
Boolean
) forces velocity streaming to stop
Returns
- (
undefined
)
streamOdometer
Starts streaming of odometer data
It uses sphero's data streaming command. User needs to listen
for the dataStreaming
or odometer
event to get the data.
Params
-
[sps=5] (
Number
) samples per second -
[remove=false] (
Boolean
) forces velocity streaming to stop
Returns
- (
undefined
)
streamVelocity
Starts streaming of velocity data
It uses sphero's data streaming command. User needs to listen
for the dataStreaming
or velocity
event to get the velocity values.
Params
-
[sps=5] (
Number
) samples per second -
[remove=false] (
Boolean
) forces velocity streaming to stop
Returns
- (
undefined
)
submitValueToInput
The Submit value To Input command takes the place of the typical user console in orbBasic and allows a user to answer an input request.
If there is no pending input request when this API command is sent, the supplied value is ignored without error.
Refer to the orbBasic language document for further information.
Params
-
val (
Number
) value to respond with -
callback (
Function
) function to be triggered with response
Returns
- (
undefined
)
version
The Version command returns a batch of software and hardware information about Sphero.
Params
-
callback (
Function
) triggered with version information
Returns
- (
undefined
)
Note: for more information about the documentation of the Sphero driver click here.
Events
disconnect
Emitted when the connection to the Sphero is closed
disconnect
Emitted when the connection to the Sphero is closed
error
Emitted when Sphero encounters an error
message
Emitted when Sphero sends a message through the serial port
notification
Emitted when Sphero sends a notification through the serial port
Values
- packet
data
Emitted when Sphero sends a notification through the serial port that contains data
Values
- data
collision
Emitted when Sphero detects a collision
collision
Emitted when Sphero receives a version response
collision
Emitted when Sphero receives getBluetoothInfo response
collision
Emitted when Sphero receives autoRecoonect response
collision
Emitted when Sphero receives power state response
collision
Emitted when Sphero receives voltage trip points response
collision
Emitted when Sphero receives level2diags response
collision
Emitted when Sphero receives packetTimes
collision
Emitted when Sphero receives chassisId response
collision
Emitted when Sphero receives read locator response
collision
Emitted when Sphero receives read rgb color response
collision
Emitted when Sphero receives perm option flags response
collision
Emitted when Sphero receives temp option flags response
collision
Emitted when Sphero receives device mode response
collision
Emitted when Sphero receives abort macro response
collision
Emitted when Sphero receives macro status response
collision
Emitted when Sphero receives battery status response
collision
Emitted when Sphero receives level 1 diasgs async msg
collision
Emitted when Sphero receives data streaming async msg
collision
Emitted when Sphero receives config block async msg
collision
Emitted when Sphero receives pre sleep warning async msg
collision
Emitted when Sphero receives macro markers async msg
collision
Emitted when Sphero receives orb basic print msg
collision
Emitted when Sphero receives orb basic ascii error msg
collision
Emitted when Sphero receives orb basic error msg
collision
Emitted when Sphero receives self level async msg
collision
Emitted when Sphero receives gyro axis exceeded async msg
Sphero Colors
Example | Name | Code | Example | Name | Code |
---|---|---|---|---|---|
aliceblue | 0xF0F8FF | antiquewhite | 0xFAEBD7 | ||
aqua | 0x00FFFF | aquamarine | 0x7FFFD4 | ||
azure | 0xF0FFFF | beige | 0xF5F5DC | ||
bisque | 0xFFE4C4 | black | 0x000000 | ||
blanchedalmond | 0xFFEBCD | blue | 0x0000FF | ||
blueviolet | 0x8A2BE2 | brown | 0xA52A2A | ||
burlywood | 0xDEB887 | cadetblue | 0x5F9EA0 | ||
chartreuse | 0x7FFF00 | chocolate | 0xD2691E | ||
coral | 0xFF7F50 | cornflowerblue | 0x6495ED | ||
cornsilk | 0xFFF8DC | crimson | 0xDC143C | ||
cyan | 0x00FFFF | darkblue | 0x00008B | ||
darkcyan | 0x008B8B | darkgoldenrod | 0xB8860B | ||
darkgray | 0xA9A9A9 | darkgreen | 0x006400 | ||
darkkhaki | 0xBDB76B | darkmagenta | 0x8B008B | ||
darkolivegreen | 0x556B2F | darkorange | 0xFF8C00 | ||
darkorchid | 0x9932CC | darkred | 0x8B0000 | ||
darksalmon | 0xE9967A | darkseagreen | 0x8FBC8F | ||
darkslateblue | 0x483D8B | darkslategray | 0x2F4F4F | ||
darkturquoise | 0x00CED1 | darkviolet | 0x9400D3 | ||
deeppink | 0xFF1493 | deepskyblue | 0x00BFFF | ||
dimgray | 0x696969 | dodgerblue | 0x1E90FF | ||
firebrick | 0xB22222 | floralwhite | 0xFFFAF0 | ||
forestgreen | 0x228B22 | fuchsia | 0xFF00FF | ||
gainsboro | 0xDCDCDC | ghostwhite | 0xF8F8FF | ||
gold | 0xFFD700 | goldenrod | 0xDAA520 | ||
gray | 0x808080 | green | 0x008000 | ||
greenyellow | 0xADFF2F | honeydew | 0xF0FFF0 | ||
hotpink | 0xFF69B4 | indianred | 0xCD5C5C | ||
indigo | 0x4B0082 | ivory | 0xFFFFF0 | ||
khaki | 0xF0E68C | lavender | 0xE6E6FA | ||
lavenderblush | 0xFFF0F5 | lawngreen | 0x7CFC00 | ||
lemonchiffon | 0xFFFACD | lightblue | 0xADD8E6 | ||
lightcoral | 0xF08080 | lightcyan | 0xE0FFFF | ||
lightgoldenrodyellow | 0xFAFAD2 | lightgreen | 0x90EE90 | ||
lightgrey | 0xD3D3D3 | lightpink | 0xFFB6C1 | ||
lightsalmon | 0xFFA07A | lightseagreen | 0x20B2AA | ||
lightskyblue | 0x87CEFA | lightslategray | 0x778899 | ||
lightsteelblue | 0xB0C4DE | lightyellow | 0xFFFFE0 | ||
lime | 0x00FF00 | limegreen | 0x32CD32 | ||
linen | 0xFAF0E6 | magenta | 0xFF00FF | ||
maroon | 0x800000 | mediumaquamarine | 0x66CDAA | ||
mediumblue | 0x0000CD | mediumorchid | 0xBA55D3 | ||
mediumpurple | 0x9370DB | mediumseagreen | 0x3CB371 | ||
mediumslateblue | 0x7B68EE | mediumspringgreen | 0x00FA9A | ||
mediumturquoise | 0x48D1CC | mediumvioletred | 0xC71585 | ||
midnightblue | 0x191970 | mintcream | 0xF5FFFA | ||
mistyrose | 0xFFE4E1 | moccasin | 0xFFE4B5 | ||
navajowhite | 0xFFDEAD | navy | 0x000080 | ||
oldlace | 0xFDF5E6 | olive | 0x808000 | ||
olivedrab | 0x6B8E23 | orange | 0xFFA500 | ||
orangered | 0xFF4500 | orchid | 0xDA70D6 | ||
palegoldenrod | 0xEEE8AA | palegreen | 0x98FB98 | ||
paleturquoise | 0xAFEEEE | palevioletred | 0xDB7093 | ||
papayawhip | 0xFFEFD5 | peachpuff | 0xFFDAB9 | ||
peru | 0xCD853F | pink | 0xFFC0CB | ||
plum | 0xDDA0DD | powderblue | 0xB0E0E6 | ||
purple | 0x800080 | red | 0xFF0000 | ||
rosybrown | 0xBC8F8F | royalblue | 0x4169E1 | ||
saddlebrown | 0x8B4513 | salmon | 0xFA8072 | ||
sandybrown | 0xF4A460 | seagreen | 0x2E8B57 | ||
seashell | 0xFFF5EE | sienna | 0xA0522D | ||
silver | 0xC0C0C0 | skyblue | 0x87CEEB | ||
slateblue | 0x6A5ACD | slategray | 0x708090 | ||
snow | 0xFFFAFA | springgreen | 0x00FF7F | ||
steelblue | 0x4682B4 | tan | 0xD2B48C | ||
teal | 0x008080 | thistle | 0xD8BFD8 | ||
tomato | 0xFF6347 | turquoise | 0x40E0D0 | ||
violet | 0xEE82EE | wheat | 0xF5DEB3 | ||
white | 0xFFFFFF | whitesmoke | 0xF5F5F5 | ||
yellow | 0xFFFF00 | yellowgreen | 0x9ACD32 |