ARDrone
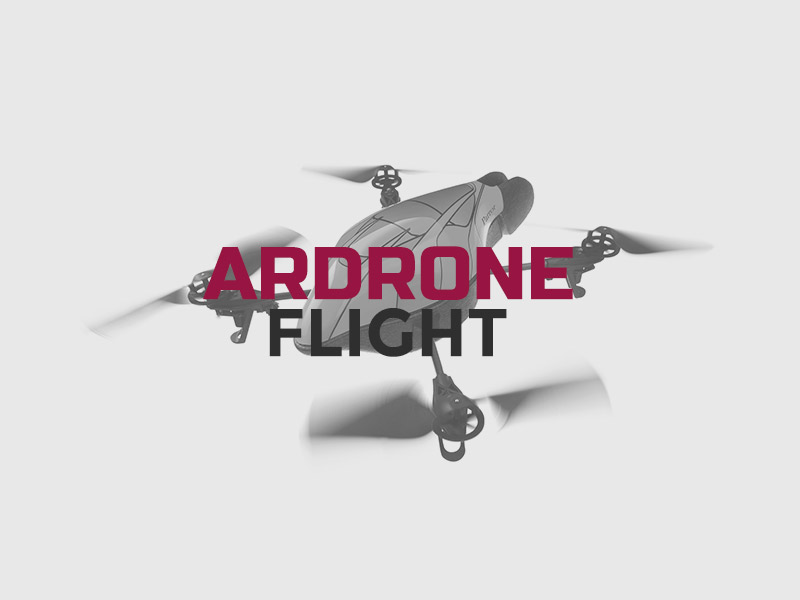
Allows user to send flight control commands to an ARDrone. Flight control commands are those used to tell the ARDrone to take off, land, and or any number of other autonomous flight manuvers.
For more information click here.
How To Connect
Communication with the ARDrone's flight control interface takes place using a WiFi connection. The ARDrone is a WiFi access point, so it normally can be the only device you connect to, without some additional effort to reconfigure the drone itself to put it into infrastructure mode.
Cylon.robot({ connections: { ardrone: { adaptor: 'ardrone', port: '192.168.1.1' } }, devices: { ardrone: { driver: 'ardrone' } }, });
How To Use
This example controls an ARDrone to take off, and then land.
var Cylon = require('cylon'); Cylon.robot({ connections: { ardrone: { adaptor: 'ardrone', port: '192.168.1.1' } }, devices: { drone: { driver: 'ardrone' } }, work: function(my) { my.drone.takeoff(); after((10).seconds(), function() { my.drone.land(); } after((15).seconds(), function() { my.drone.stop(); } } }).start();
Commands
takeoff
Sets the internal 'fly' state to 'true'.
Params
-
callback (
Function
) function to be invoked once the drone is hovering
Returns
-
( Boolean
null
)
land
Sets the internal 'fly' state to 'false'.
Params
-
callback (
Function
) function to be invoked when the drone has landed
Returns
-
( Boolean
null
)
stop
Sets all drone movements to 0.
This effectively makes it hover in place.
Returns
- (
null
)
up
Makes the drone gain altitude.
Params
-
speed (
Number
) a 0-1 value for how fast the drone should climb
Returns
- (
Number
)
down
Makes the drone lose altitude.
Params
-
speed (
Number
) a 0-1 value for how fast the drone should fall
Returns
- (
Number
)
left
Makes the drone bank to the left.
Controls the roll and horizontal movement using the camera as a reference point.
Params
-
speed (
Number
) a 0-1 value for how fast the drone moves left
Returns
- (
Number
)
right
Makes the drone bank to the right.
Controls the roll and horizontal movement using the camera as a reference point.
Params
-
speed (
Number
) a 0-1 value for how fast the drone moves right
Returns
- (
Number
)
front
Makes the drone bank forwards.
Controls the pitch and horizontal movement using the camera as a reference point.
Params
-
speed (
Number
) a 0-1 value for how fast the drone moves forward
Returns
- (
Number
)
back
Makes the drone bank backwards.
Controls the pitch and horizontal movement using the camera as a reference point.
Params
-
speed (
Number
) a 0-1 value for how fast the drone moves backwards
Returns
- (
Number
)
clockwise
Makes the drone spin in a clockwise direction
Params
-
speed (
Number
) a 0-1 value for how fast the drone should spin
Returns
- (
Number
)
counterClockwise
Makes the drone spin in a counter-clockwise direction
Params
-
speed (
Number
) a 0-1 value for how fast the drone should spin
Returns
- (
Number
)
calibrate
Tells the drone to calibrate a device
Params
-
deviceNum (
Number
) the device the drone should calibrate
Returns
- (
null
)
config
Tells the drone to set a configuration value
Params
-
key (
String
) the config value to set -
value (
String
) the value to set it to -
callback (
Function
) a callback to be triggered when it's done
Returns
- (
null
)
animate
Performs a pre-programmed flight sequence for a given duration.
Params
-
animation (
String
) the animation to perform -
duration (
Number
) the duration to perform the animation
Returns
- (
null
)
animateLeds
Performs a pre-programmed LED animation sequence for a given duration, at a given frequency.
Params
-
animation (
String
) the animation to perform -
hz (
Number
) the frequency to perform the animation at -
duration (
Number
) the duration to perform the animation
Returns
- (
null
)
disableEmergency
Resets the emergency state of the drone.
It does this by setting the emergency REF bit to 1
until
navdata.droneState.emergencyLanding
is 0
.
This reccovers a drone that has flipped over and shows red lights to be flyable again (with green LEDs).
It is also done implicitly when creating a new high-level client.
Returns
- (
null
)
forward
Makes the drone bank forwards.
Params
-
speed (
Number
) a 0-1 value for how fast the drone moves forward
Returns
- (
Number
)
frontFlip
Tells the drone to do as many front flips as it can in duration
.
Params
-
duration (
Number
) the duration to do front-flips for
Returns
- (
null
)
backFlip
Tells the drone to do as many back flips as it can in duration
.
Params
-
duration (
Number
) the duration to do back-flips for
Returns
- (
null
)
leftFlip
Tells the drone to do as many left flips as it can in duration
.
Params
-
duration (
Number
) the duration to do left-flips for
Returns
- (
null
)
rightFlip
Tells the drone to do as many right flips as it can in duration
.
Params
-
duration (
Number
) the duration to do right-flips for
Returns
- (
null
)
wave
Tells the drone to do as many waves as it can in duration
.
Params
-
duration (
Number
) the duration to wave for
Returns
- (
null
)
getPngStream
Requests a stream of PNGs from the ARDrone's camera.
PNGs are emitted through the data
event on the returned object as they
become ready.
Returns
- (
Object
) an object that streams PNGs from the drone's camera
hover
Tells the drone to hover in place.
Params
-
duration (
Number
) the duration to wave for
Returns
- (
null
)
ftrim
Tells the drone to perform horizontal trimming.
Returns
- (
null
)