Pinoccio LED
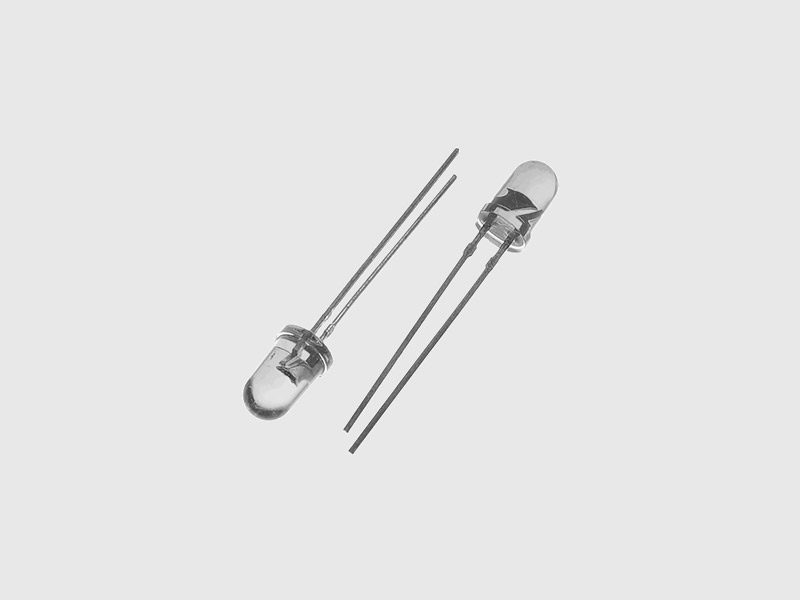
For more information click here.
How To Connect
Install the module with: npm install cylon-pinoccio
var Cylon = require('cylon'); Cylon.robot({ connections: { pinoccio: { adaptor: 'pinoccio', token: 'XXX', troop: '1', scout: '1' } }, devices: { led: { driver: 'pinoccio-led' } }, });
How To Use
var Cylon = require('cylon'); Cylon.robot({ connections: { pinoccio: { adaptor: 'pinoccio', token: 'XXX', troop: '1', scout: '1' } }, devices: { led: { driver: 'pinoccio-led' } }, work: function(my) { var isOn = false; my.led.blink(127, 127, 127); every((1).second(), function() { if (isOn === true) { isOn = false; my.led.turnOff(); } else { isOn = true; my.led.turnOn(); } }); } }).start();
Commands
turnOn
Turns the LED on.
Returns
- (
undefined
)
turnOff
Turns the LED off.
Returns
- (
undefined
)
red
Turns the LED red.
Returns
- (
undefined
)
green
Turns the LED green.
Returns
- (
undefined
)
blue
Turns the LED blue.
Returns
- (
undefined
)
cyan
Turns the LED cyan.
Returns
- (
undefined
)
purple
Turns the LED purple.
Returns
- (
undefined
)
magenta
Turns the LED magenta.
Returns
- (
undefined
)
yellow
Turns the LED yellow.
Returns
- (
undefined
)
orange
Turns the LED orange.
Returns
- (
undefined
)
white
Turns the LED white.
Returns
- (
undefined
)
torch
Enables the LED's torch mode
Returns
- (
undefined
)
hex
Sets the LED's color based on a provided hex color
Params
-
value (
String
) the hex string of the color to use
Returns
- (
undefined
)
rgb
Sets the LED's color based on a provided RGB color
Params
-
r (
String
) red value -
g (
String
) green value -
b (
String
) blue value
Returns
- (
undefined
)
saveTorch
Saves the LED's torch color based on a provided RGB color
Params
-
r (
String
) red value -
g (
String
) green value -
b (
String
) blue value
Returns
- (
undefined
)
blink
Blinks the LED with the provided RGB pattern
Params
-
r (
String
) red value -
g (
String
) green value -
b (
String
) blue value
Returns
- (
undefined
)