Sphero
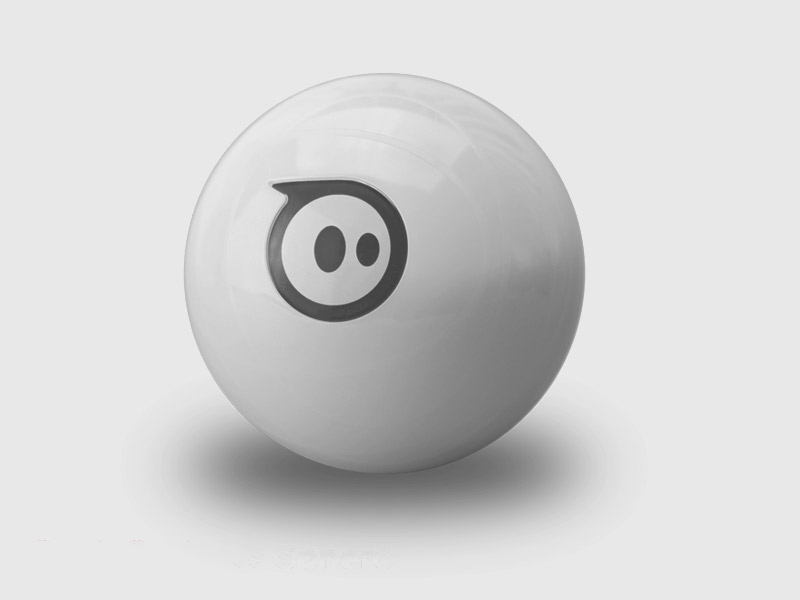
Repository| Issues
Sphero is a sophisticated and programmable robot housed in a polycarbonate sphere shell.
The cylon-sphero adaptor makes it easy to interact with Sphero using Node.js. Once you have your Sphero setup and connected to your computer, you can start writing code to make Sphero move, change direction, speed and colors, or detect Sphero events and execute some code when they occur.
To learn more about the Sphero, click here.
How to Install
Install the module with:
$ npm install cylon cylon-sphero
How to Use
Example of a simple program that makes the Sphero roll.
var Cylon = require('cylon'); Cylon.robot({ connections: { sphero: { adaptor: 'sphero', port: '/dev/rfcomm0' } }, devices: { sphero: { driver: 'sphero' } }, work: function(my) { every((1).second(), function() { my.sphero.roll(60, Math.floor(Math.random() * 360)); }); } }).start();
How to Connect
OSX
In order to allow Cylon.js running on your Mac to access the Sphero, go to "Bluetooth > Open Bluetooth Preferences > Sharing Setup" and make sure that "Bluetooth Sharing" is checked.
Thank you to @kopipejst for the above connnection info.
First pair your computer and Sphero. You can do this using bluetooth preferences. (Sphero won't stay connected)
Find out serial port address by running this command:
ls /dev/tty.Sphero*
The port will look something like this:
/dev/tty.Sphero-BBP-AMP-SPP
Now you are ready to run the example code, be sure to update this line with the correct port:
connections: { sphero: { adaptor: "sphero", port: "/dev/tty.Sphero-BBP-AMP-SPP" } },
Ubuntu
Connecting to the Sphero from Ubuntu or any other Linux-based OS can be done entirely from the command line using CylonJS CLI commands. Here are the steps.
Find the address of the Sphero, by using:
gort bluetooth scan
Pair to Sphero using this command (substituting the actual address of your Sphero):
gort bluetooth pair <address>
Connect to the Sphero using this command (substituting the actual address of your Sphero):
gort bluetooth connect <address>
Windows
You should be able to simply pair your Sphero using your normal system tray applet for Bluetooth, and then connect to the COM port that is bound to the device, such as COM3
.
Compatibility
The cylon-sphero module is currently compatible with Node.js versions 0.10.x thru 5.x.
In order to install it with Node.js 5.x+, you will need to have g++ v4.8 or higher.
How To Calibrate Sphero
You might want to calibrate the orientation of the Sphero so that it is pointed 'forward'. There are 2 functions that have been added to the Sphero driver to help with this.
Call startCalibration()
to put the Sphero into 'calibration mode' by turning on the tail LED and turning off the auto-stablization. You can now manually turn the Sphero to so the tail LED is pointed to the rear of the direction in which you want the Sphero to go.
Call finishCalibration()
to turn off 'calibration mode' by turning off the tail LED and turning back on the auto-stablization. Whichever direction that the tail LED was pointed, is now the rear direction for the Sphero.
Drivers
There is only one driver for the Sphero. Click on the image below: