BlinkM
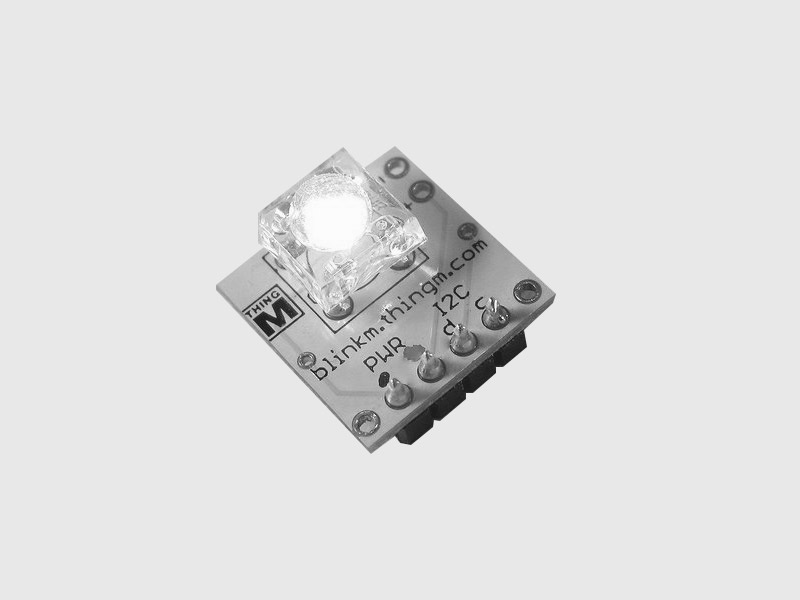
Allows user to control BlinkM RGB LED via i2c interface.
For more information click here.
How To Connect
Install the module with npm install cylon-i2c
Cylon.robot({ connections: { arduino: { adaptor: 'firmata', port: '/dev/ttyACM0' } }, devices: { led: { driver: 'led', pin: 13 } }, });
How To Use
Example, using a BlinkM LED with an Arduino:
"use strict"; var Cylon = require("cylon"); Cylon.robot({ connections: { arduino: { adaptor: "firmata", port: "/dev/ttyACM0" } }, devices: { blinkm: { driver: "blinkm" } }, work: function(my) { my.blinkm.stopScript(); my.blinkm.getFirmware(function(err, version) { console.log(err || "Started BlinkM version " + version); }); my.blinkm.goToRGB(0, 0, 0); my.blinkm.getRGBColor(function(err, data) { console.log(err || "Starting Color: ", data); }); every((2).seconds(), function() { my.blinkm.getRGBColor(function(err, data) { console.log(err || "Current Color: ", data); }); my.blinkm.fadeToRandomRGB(128, 128, 128); }); } }).start();
Commands
goToRGB
Sets the color of the BlinkM to the specified combination of RGB values.
Params
-
r (
Number
) red value, 0-255 -
g (
Number
) green value, 0-255 -
b (
Number
) blue value, 0-255 -
callback (
Function
) function to invoke when complete
Returns
- (
undefined
)
fadeToRGB
Fades the color of the BlinkM to the specified combination of RGB values.
Params
-
r (
Number
) red value, 0-255 -
g (
Number
) green value, 0-255 -
b (
Number
) blue value, 0-255 -
callback (
Function
) function to invoke when complete
Returns
- (
undefined
)
fadeToHSB
Fades the color of the BlinkM to the specified combination of HSB values.
Params
-
h (
Number
) hue value, 0-359 -
s (
Number
) saturation value, 0-100 -
b (
Number
) brightness value, 0-100 -
callback (
Function
) function to invoke when complete
Returns
- (
undefined
)
fadeToRandomRGB
Fades the color of the BlinkM to a random combination of RGB values.
Params
-
r (
Number
) red value, 0-255 -
g (
Number
) green value, 0-255 -
b (
Number
) blue value, 0-255 -
cb (
Function
) function to invoke when complete
Returns
- (
undefined
)
fadeToRandomHSB
Fades the color of the BlinkM to a random combination of HSB values.
Params
-
h (
Number
) hue value, 0-359 -
s (
Number
) saturation value, 0-100 -
b (
Number
) brightness value, 0-100 -
cb (
Function
) function to invoke when complete
Returns
- (
undefined
)
playLightScript
Plays a light script for the BlinkM.
Available scripts are available in the BlinkM datasheet.
A repeats
value of 0
causes the script to execute until the the
#stopScript
command is called.
Params
-
id (
Number
) light script to play -
repeats (
Number
) whether the script should repeat -
startAtLine (
Number
) which line in the light script to start at -
cb (
Function
) function to invoke when complete
Returns
- (
undefined
)
stopScript
Stops the currently executing BlinkM light script.
Params
-
cb (
Function
) function to invoke when complete
Returns
- (
undefined
)
setFadeSpeed
Sets the fade speed for the BlinkM
Params
-
speed (
Number
) how fast colors should fade (1-255) -
cb (
Function
) function to invoke when complete
Returns
- (
undefined
)
setTimeAdjust
Sets a time adjust for the BlinkM.
This affects the duration of scripts.
Params
-
time (
Number
) an integer between -128 and 127. 0 resets the time. -
cb (
Function
) function to invoke when complete
Returns
- (
undefined
)
getRGBColor
Gets the RGB values for the current BlinkM color.
Yields an array in the form [r, g, b]
, each a 0-255 integer.
Params
-
cb (
Function
) function to invoke when complete
Returns
- (
undefined
)
getAddress
Returns a string describing the current I2C address being used.
Params
-
cb (
Function
) function to invoke when complete
Returns
- (
undefined
)
setAddress
Sets an address to the BlinkM driver
Params
-
address (
Number
) I2C address to set -
cb (
Function
) function to invoke when complete
Returns
- (
undefined
)
getFirmware
Returns a string describing the I2C firmware version being used
Params
-
cb (
Function
) function to invoke when complete
Returns
- (
undefined
)
Circuit
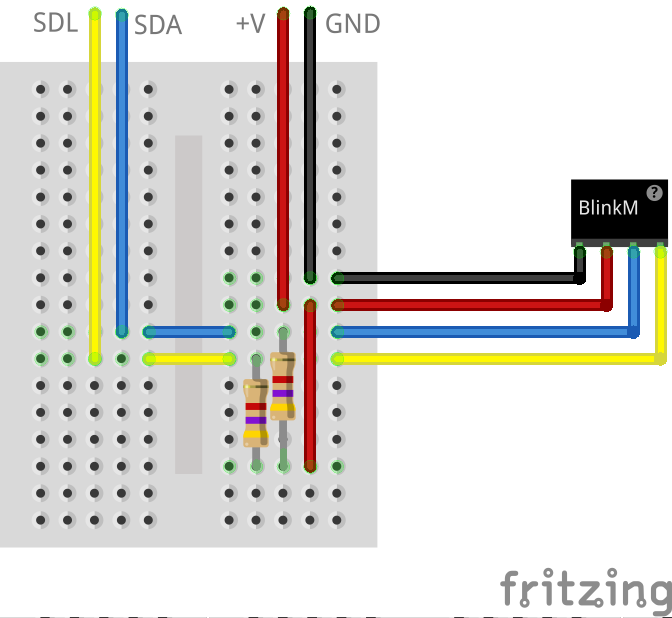