Tessel Servo
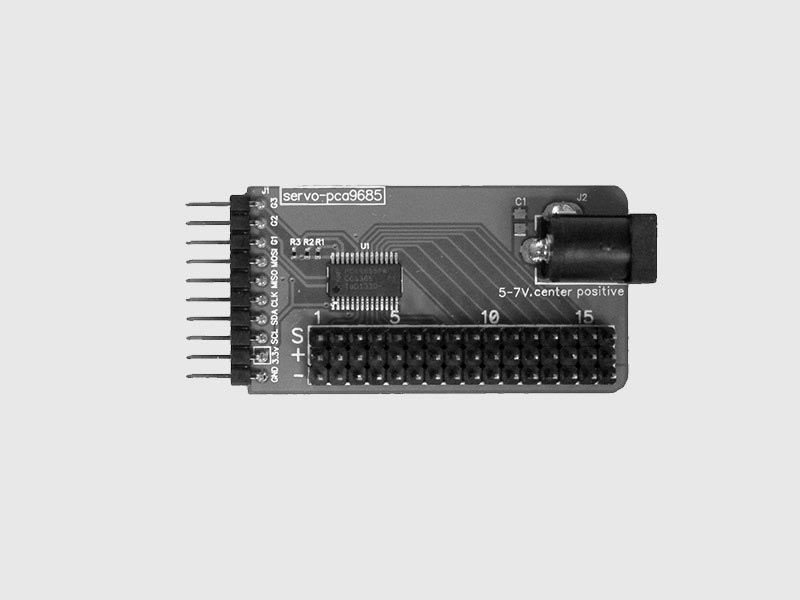
Control up to 16 hobbyist/RC servos.
Make it move! Control locks, wheels, cords, or anything else you can think of.
- Standard PWM output is compatible with servos of all sizes
- Takes a separate barrel jack power connection (included with US shipments)
- Comes with an ES3001 YinYan Servo and a 5V external power jack (US-style plug)
- Can also be used as an LED driver.
For more info visit here.
How To Connect
Cylon.robot({ connections: { tessel: { adaptor: 'tessel', port: 'A' } }, devices: { servo: { driver: 'servo-pca9685' } }, });
How To Use
Example using a Direct Pin.
var Cylon = require('cylon'); Cylon.robot({ connections: { tessel: { adaptor: 'tessel', port: 'A' } }, devices: { servo: { driver: 'servo-pca9685' } }, work: function(my) { var servo1 = 1; // We have a servo plugged in at position 1 var position = 0; // Target position of the servo between 0 (min) and 1 (max). my.servo.on('error', function (err) { console.log(err) }); // Set the minimum and maximum duty cycle for servo 1. // If the servo doesn't move to its full extent or stalls out // and gets hot, try tuning these values (0.05 and 0.12). // Moving them towards each other = less movement range // Moving them apart = more range, more likely to stall and burn out my.servo.configure(servo1, 0.05, 0.12, function () { every((0.5).seconds(), function() { console.log('Position (in range 0-1):', position); // Set servo #1 to position pos. my.servo.move(servo1, position); // Increment by 10% (~18 deg for a normal servo) position += 0.1; if (position > 1) { position = 0; // Reset servo position } }); }); } }).start();
Commands
servo.configure( whichServo, minPWM, maxPWM, callback() )
Sets the PWM max and min for the specified servo.
servo.move( whichServo, positionOrSpeed, callback() )
positionOrSpeed is a value between 0 and 1. On a normal servo, this value is the position to move to as a percent of the total available rotational range. On a continuous rotation servo, this value represents the rotation speed: 0 is fast in one direction, 1 is fast in the other direction, and 0.5 is stopped.
servo.read( whichServo, callback() )
Reads the current approximate position target for the specified servo.
servo.setDutyCycle( whichServo, on, callback() )
Sets the duty cycle for the specified servo. on is duty cycle uptime, range from 0-1.
servo.setModuleFrequency( Hertz, callback() )
Sets the PWM frequency in Hz for the PCA9685 chip.
Events
'error'
Emitted upon error.
'ready'
Emitted upon first successful communication between the Tessel and the module.