Joysticks
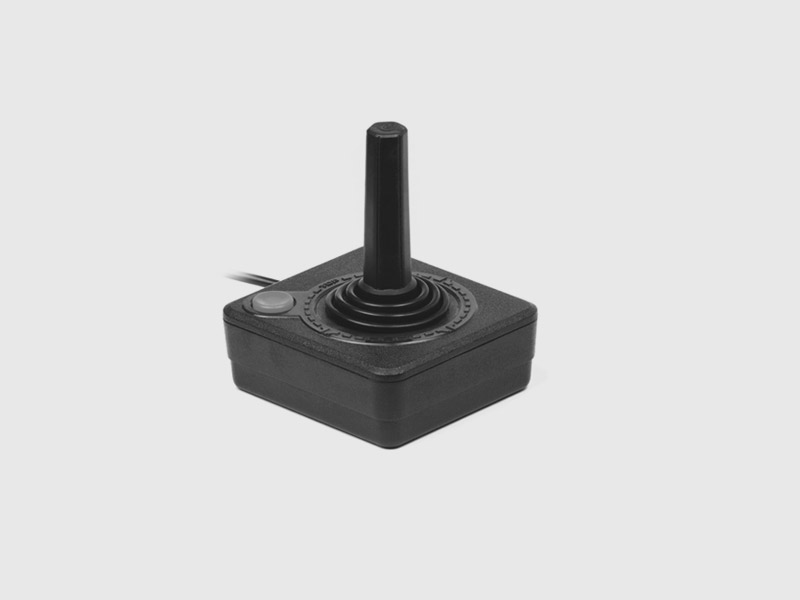
Repository| Issues
You can use Cylon.js with a DualShock 3 controller, an Xbox 360 controller, or any other Simple DirectMedia Layer (SDL) compatible USB joystick or game controller.
For more info about SDL, click here.
How to Install
Installing Cylon.js with Joystick support is pretty easy.
$ npm install cylon cylon-joystick
Note
- OS X does not provide native support for Xbox 360 controllers. As such, a third-party driver is required.
- If you're using a PS3 controller and want to communicate with it over USB, plug it in and then press the PlayStation button to make sure it's connected.
How to Use
var Cylon = require('cylon'); Cylon.robot({ connections: { joystick: { adaptor: 'joystick' } }, devices: { controller: { driver: 'dualshock-3' } }, work: function(my) { ["square", "circle", "x", "triangle"].forEach(function(button) { my.controller.on(button + ":press", function() { console.log("Button " + button + " pressed."); }); my.controller.on(button + ":release", function() { console.log("Button " + button + " released."); }); }); my.controller.on("left_x:move", function(pos) { console.log("Left Stick - X:", pos); }); my.controller.on("right_x:move", function(pos) { console.log("Right Stick - X:", pos); }); my.controller.on("left_y:move", function(pos) { console.log("Left Stick - Y:", pos); }); my.controller.on("right_y:move", function(pos) { console.log("Right Stick - Y:", pos); }); } }).start();
How to Connect
Plug your USB joystick or game controller into your USB port. If your device is supported by SDL, you are now ready.
Custom joysticks
If you don't have one of the joysticks we support natively, or want to make changes to the configuration, cylon-joystick
supports custom bindings.
To use a custom joystick with Cylon, simply supply the joystick bindings file when you're describing the device:
var Cylon = require('cylon'); var config = __dirname + "/controller.json" Cylon.robot({ connections: { joystick: { adaptor: 'joystick' } }, devices: { controller: { driver: "joystick", config: config } }, work: function(my) { // your custom mappings will be reflected here as events } }).start();
A joystick bindings file needs to contain the device's productID
and vendorID
, as this is how cylon-joystick will find the appropriate device.
For an example of what a bindings file should look like, here is the Xbox 360 controller bindings file we use.
cylon-joystick-explorer
cylon-joystick
includes the cylon-joystick-explorer
binary.
It's useful for figuring out what compatible gamepads you have connected, as well as making it easier to generate custom bindings JSON files.
For best use, install cylon-joystick
globally:
$ npm install -g cylon-joystick
Then just run the command:
$ cylon-joystick-explorer
Drivers
Available drivers for the joystick platform are listed below: