Direct Pin
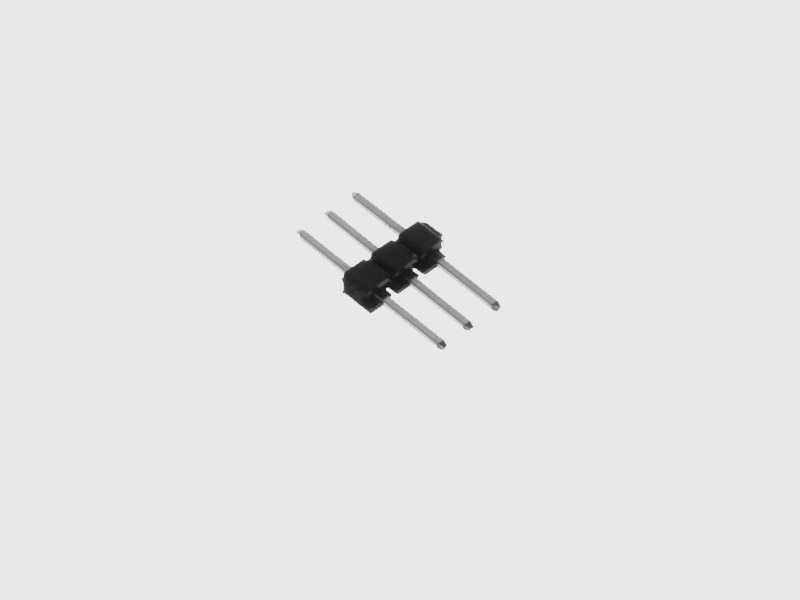
This a prototyping driver.
How To Connect
Install the module with npm install cylon-gpio
Cylon.robot({ connections: { arduino: { adaptor: 'firmata', port: '/dev/ttyACM0' } }, devices: { pin: { driver: 'direct-pin', pin: 13 } }, });
How To Use
Example using a Direct Pin.
var Cylon = require('cylon'); Cylon.robot({ connections: { arduino: { adaptor: 'firmata', port: '/dev/ttyACM0' } }, devices: { pin: { driver: 'direct-pin', pin: 13 } }, work: function(my) { var value = 0; every((1).second(), function() { my.pin.digitalWrite(value); value = (value == 0) ? 1 : 0; }); } }).start();
Commands
digitalWrite
Writes a digital value to the pin
Params
-
value (
Number
) value to write to the pin -
[callback] (
Function
) - (err, val) triggers when write is complete
Returns
- (
undefined
)
analogWrite
Writes an analog value to the pin
Params
-
value (
Number
) value to write to the pin -
[callback] (
Function
) - (err, val) triggers when write is complete
Returns
- (
undefined
)
digitalRead
Reads the value from the pin
Triggers the provided callback when the pin state has been read.
Params
-
callback (
Function
) triggered when the pin state has been read
Returns
- (
undefined
)
analogRead
Reads the value from the pin
Triggers the provided callback when the pin state has been read.
Params
-
callback (
Function
) triggered when the pin state has been read
Returns
- (
undefined
)
servoWrite
Writes a servo value to the pin
Params
-
angle (
Number
) angle value to write to the pin -
[callback] (
Function
) - (err, val) triggers when write is complete
Returns
- (
undefined
)
pwmWrite
Writes a PWM value to the pin
Params
-
value (
Number
) value to write to the pin -
[callback] (
Function
) - (err, val) triggers when write is complete
Returns
- (
undefined
)