MQTT
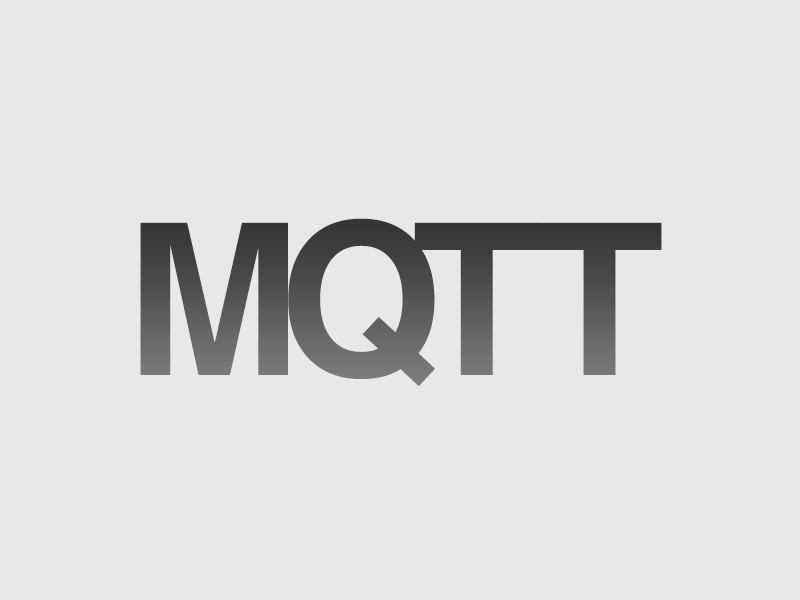
The Cylon driver for the MQTT adaptor.
How To Connect
var Cylon = require('cylon'); Cylon.robot({ connections: { server: { adaptor: 'mqtt', host: 'mqtt://localhost:1883' } }, work: function(my) { my.server.subscribe('hello'); my.server.on('message', function (topic, data) { console.log(topic + ": " + data); }); every((1).seconds(), function() { console.log("Saying hello..."); my.server.publish('hello', 'hi there'); }); } }).start();
How To Use
The following is an example of how to make an arduino blink with MQTT.
var Cylon = require('cylon'); Cylon.robot({ connections: { mqtt: { adaptor: 'mqtt', host: 'mqtt://localhost:1883' }, firmata: { adaptor: 'firmata', port: '/dev/ttyACM0' } }, devices: { toggle: { driver: 'mqtt', topic: 'toggle', adaptor: 'mqtt' }, led: { driver: 'led', pin: '13', adaptor: 'firmata' }, }, work: function(my) { my.toggle.on('message', function(data) { console.log("Message on 'toggle': " + data); my.led.toggle(); }); every((1).second(), function() { console.log("Toggling LED."); my.toggle.publish('toggle'); }); } }).start();
Commands
publish
Publishes a new message on the Driver's topic
Params
-
data (
Object
,String
) the data to publish to the topic -
[opts] (
Object
) - publish options, includes: {Number} qos - qos level to publish on {Boolean} retain - whether or not to retain the message
Returns
- (
undefined
)
Events
message
Emitted when the MQTT client receives a new message on the Driver's topic
Values
- message