Intel IoT Analytics
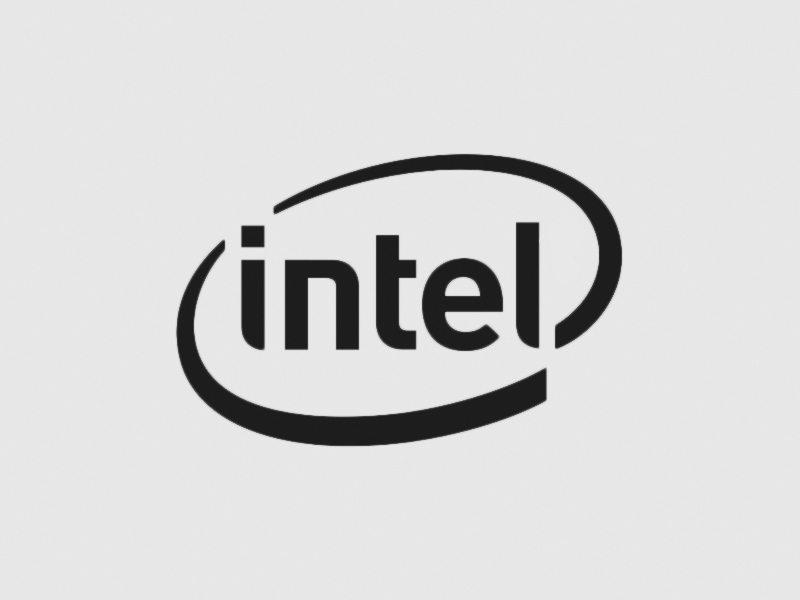
Intel IoT Analytics is a cloud-based system designed for the Internet-of-Things (IoT). It includes resources for Intel Galileo/Edison device developers and provides easily acquirable data and analysis.
For more info on the Intel IoT Analytics platform click here.
How To Connect
"use strict"; var Cylon = require("cylon"); Cylon.robot({ connections: { "iot-analytics": { adaptor: "intel-iot-analytics", username: "username", password: "password" } }, devices: { iot: { driver: "iot" } }, work: function(my) { var aId = "f5dbea6a-7115-4f77-9919-63c23ec83d9b"; var device = { "deviceId": "raspi-01", "gatewayId": "24-a5-80-21-5b-29", "name": "Raspberry Pi 01", "tags": ["raspi", "raspberry-pi", "cylon"], "loc": [ 45.5434085, -122.654422, 124.3 ], "attributes": { "vendor": "raspberry inc", "platform": "ARM", "os": "linux" } }; console.log("Connecting to IoT analytics:"); my.iot.createDevice(aId, device, function(err, res) { console.log("error:", err); console.log("New Device:", res); }); } }).start();
How To Use
"use strict"; var Cylon = require("cylon"); Cylon.robot({ connections: { "iot-analytics": { adaptor: "intel-iot-analytics", username: "username", password: "password" } }, devices: { iot: { driver: "iot" } }, work: function(my) { var aId = "f5dbea6a-7115-4f77-9919-63c23ec83d9b"; console.log("Connecting to IoT analytics:"); my.iot.device(aId, "arduino-uno-id1", function(err, device) { console.log("error:", err); console.log("Device details:", device); }); } }).start();
Commands
accountInfo
Get account details
GET /accounts/
Params
-
accountId (
String
) The account to get details for -
callback (
Function
) called on complete: function(err, res) {}
Returns
- (
undefined
)
activationCode
Retrieve account activation code
GET /accounts/
Params
-
accountId (
String
) The account to get details for -
callback (
Function
) called on complete: function(err, res) {}
Returns
- (
undefined
)
refreshActivationCode
Forces the renewal of the account activation code
PUT /accounts/
Params
-
accountId (
String
) The account to get details for -
callback (
Function
) called on complete: function(err, res) {}
Returns
- (
undefined
)
devices
Get a list of devices
GET /accounts/
Params
-
accountId (
String
) The account the devices belong to -
[filters] (
String
) Filter the list of devices -
callback (
Function
) called on complete: function(err, res) {}
Returns
- (
undefined
)
device
Get device details
GET /accounts/
Params
-
accountId (
String
) The account the device belongs to -
deviceId (
String
) The Id of the device -
callback (
Function
) called on complete: function(err, res) {}
Returns
- (
undefined
)
createDevice
Creates a new device
POST /accounts/
Params
-
accountId (
String
) The account to create the new device in -
device (
Object
) The device -
callback (
Function
) called on complete: function(err, res) {}
Returns
- (
undefined
)
updateDevice
Update an existing device
PUT /accounts/
Params
-
accountId (
String
) The account the device belongs to -
deviceId (
String
) The device to be updated -
device (
Object
) The device details -
cb (
Function
) called on complete: function(err, res) {}
Returns
- (
undefined
)
activateDevice
Activate a existing device
PUT /accounts/
Params
-
accountId (
String
) The account the device belongs to -
deviceId (
String
) The device to be updated -
code (
Object
) device activation code -
cb (
Function
) called on complete: function(err, res) {}
Returns
- (
undefined
)
deleteDevice
Delete a device
DELETE /accounts/
Params
-
accountId (
String
) The account the device belongs to -
deviceId (
String
) The device to be deleted -
callback (
Function
) called on complete: function(err, res) {}
Returns
- (
undefined
)
addComponent
Add a componenent to a device
POST /accounts/
Params
-
aId (
String
) The account the device belongs to -
dId (
String
) The device to be added to -
dToken (
String
) token of the device to update -
com (
Object
) The component to be added -
callback (
Function
) called on complete: function(err, res) {}
Returns
- (
undefined
)
removeComponent
Delete a componenent from a device
DELETE /accounts/
Params
-
aId (
String
) The account the device belongs to -
dId (
String
) The device the component belongs to -
cId (
String
) The component to be deleted -
callback (
Function
) called on complete: function(err, res) {}
Returns
- (
undefined
)
getAllTags
List all tags for devices
GET /accounts/
Params
-
aId (
String
) The account the device belongs to -
callback (
Function
) called on complete: function(err, res) {}
Returns
- (
undefined
)
getAllAttrs
List all attributes for devices
GET /accounts/
Params
-
aId (
String
) The account the device belongs to -
callback (
Function
) called on complete: function(err, res) {}
Returns
- (
undefined
)
getAllComponents
List all componenets for an account
GET /accounts/
Params
-
aId (
String
) The account the device belongs to -
callback (
Function
) called on complete: function(err, res) {}
Returns
- (
undefined
)
component
Get component details
GET /accounts/
Params
-
aId (
String
) The account the device belongs to -
cId (
String
) The component ID -
cb (
Function
) called on complete: function(err, res) {}
Returns
- (
undefined
)
createComponent
Creates a new custom component
POST /accounts/
Params
-
accountId (
String
) The account we want to add the new component to -
component (
Object
) component data -
callback (
Function
) called on complete: function(err, res) {}
Returns
- (
undefined
)
updateComponent
Update a custom component
POST /accounts/
Params
-
aId (
String
) The account we want to add the new component to -
cId (
String
) The component to be modified -
component (
Object
) the component data -
callback (
Function
) called on complete: function(err, res) {}
Returns
- (
undefined
)
submitData
Submit data to a devices component
POST /data/
Params
-
aId (
String
) The account we want to add the new component to -
dId (
String
) The device we want to add data to -
dToken (
String
) token for device we want to add data to -
data (
Array
) Array of objects with component data details -
callback (
Function
) called on complete: function(err, res) {}
Returns
- (
undefined
)
retrieveData
Retrieve data from device
POST /accounts/
Params
-
aId (
String
) the account we want to add the new component to -
filters (
Object
) filters for the search -
callback (
Function
) called on complete: function(err, res) {}
Returns
- (
undefined
)