BMP180
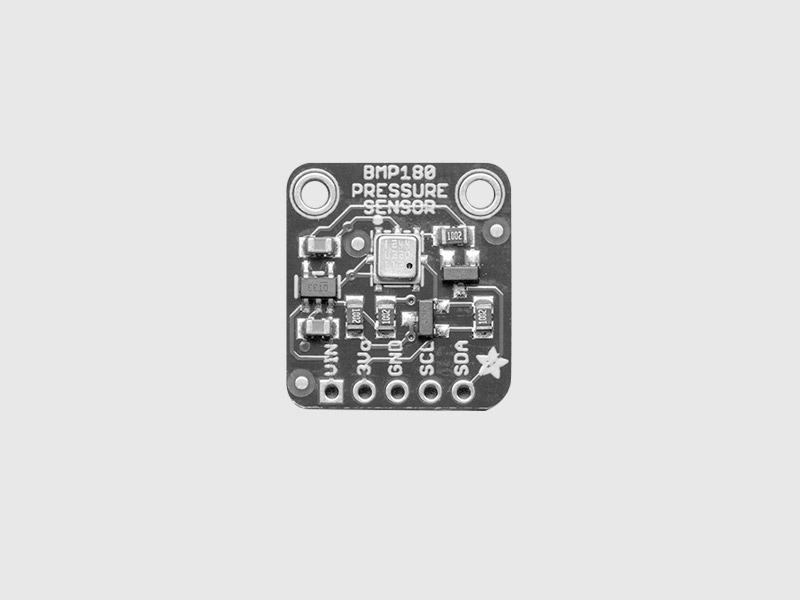
This is a breakout board for the Bosch BMP180 high-precision, low-power digital barometer. The BMP180 offers a pressure measuring range of 300 to 1100 hPa with an accuracy down to 0.02 hPa in advanced resolution mode. It's based on piezo-resistive technology for high accuracy, ruggedness and long term stability.
For more information click here.
How To Connect
Cylon.robot({ connections: { raspi: { adaptor: 'raspi' } }, devices: { bmp180: { driver: 'bmp180' } }, });
How To Use
This example lets you get the temperature, pressure and altitude.
var Cylon = require('cylon'); Cylon.robot({ connections: { raspi: { adaptor: 'raspi' } }, devices: { bmp180: { driver: 'bmp180' } }, work: function(my) { my.bmp180.getTemperature(function(err, val) { if (err) { console.log(err); return; } console.log("getTemperature call:"); console.log("\tTemp: " + val.temp + " C"); }); after((1).second(), function() { my.bmp180.getPressure(1, function(err, val) { if (err) { console.log(err); return; } console.log("getPressure call:"); console.log("\tTemperature: " + val.temp + " C"); console.log("\tPressure: " + val.press + " Pa"); }); }); after((2).seconds(), function() { my.bmp180.getAltitude(1, null, function(err, val) { if (err) { console.log(err); return; } console.log("getAltitude call:"); console.log("\tTemperature: " + val.temp + " C"); console.log("\tPressure: " + val.press + " Pa"); console.log("\tAltitude: " + val.alt + " m"); }); }); } }).start();
Commands
getPressure
Gets the value of the pressure in Pascals.
Since temperature is also calculated to determine pressure, it returns the temperature as well.
Params
-
mode (
Number
) mode to use -
callback (
Function
) function to be invoked with data
Returns
- (
undefined
)
getTemperature
Gets the value of the temperature in degrees Celsius.
Params
-
callback (
Function
) function to be invoked with data
Returns
- (
undefined
)
getAltitude
Calculates the altitude from the pressure and temperature.
Since temperature and pressure are calculated to determine altitude, it returns all three.
Params
-
mode (
Number
) which mode to use -
seaLevelPressure (
Number
) the pressure at sea level -
callback (
Function
) function to be invoked with data
Returns
- (
undefined
)
Circuit
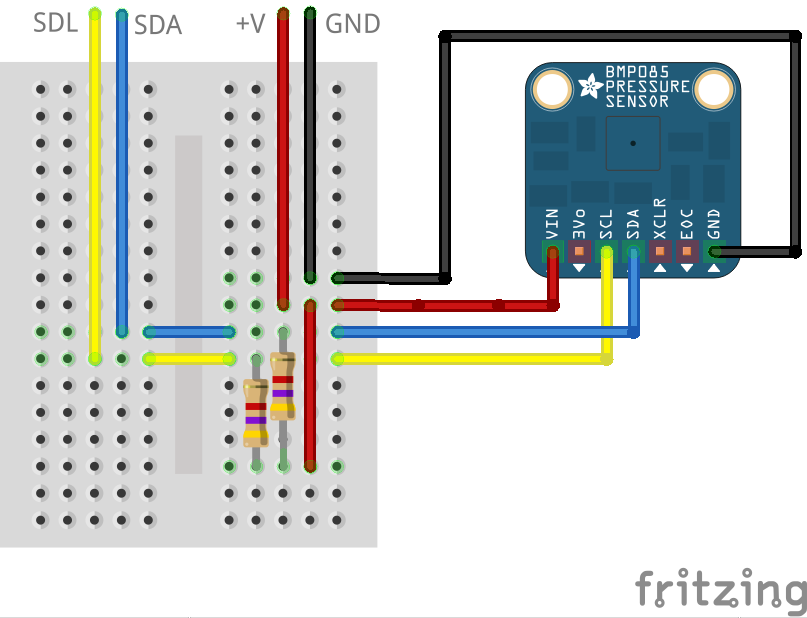