RGB LED
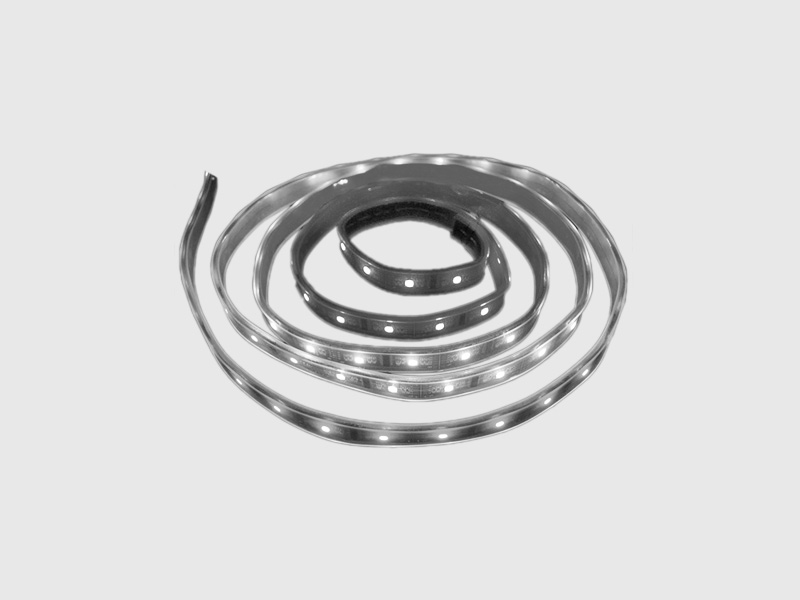
Allows user to interact with LED strips, with each light accepting RBG values through PWM (Pulse Width Modulation)
For more information click here.
How To Connect
var Cylon = require('cylon'); Cylon.robot({ connections: { edison: { adaptor: 'intel-iot' } }, devices: { leds: { driver: 'rgb-led', redPin: 3, greenPin: 5, bluePin: 6 }, }, });
How To Use
This example turns an LED on and off.
var Cylon = require('cylon'); Cylon.robot({ connections: { edison: { adaptor: 'intel-iot' } }, devices: { leds: { driver: 'rgb-led', redPin: 3, greenPin: 5, bluePin: 6 }, }, work: function(my) { var color; every((1).second(), function() { if (color == "ff0000") { color = "00ff00" } else { color = "ff0000" }; my.leds.setRGB(color); }); } }).start();
Commands
setRGB
Sets the RGB LED to a specific color
Params
-
hex (
Number
) value for the LED e.g. 0xff00ff -
callback (
Function
) to be triggered when complete
Returns
- (
undefined
)
isOn
Returns whether or not the RGB LED is currently on
Params
-
callback (
Function
) function to invoke with isOn value
Returns
- (
Boolean
) whether or not the LED is currently on