Servo
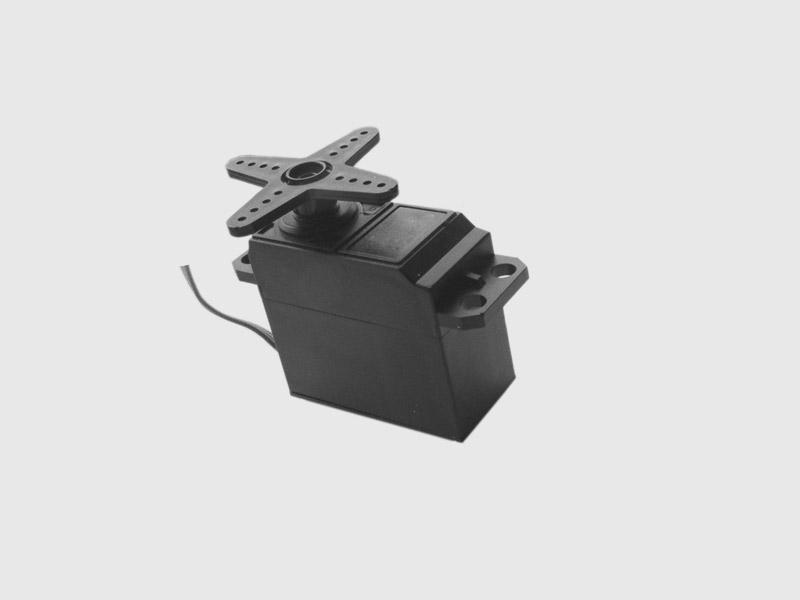
Provides an interface for making servos easy to work and interact with from Cylon.js.
For more information click here.
How To Connect
Cylon.robot({ connections: { arduino: { adaptor: 'firmata', port: '/dev/ttyACM0' } }, devices: { servo: { driver: 'servo', pin: 3 } }, });
How To Use
This example moves a servo from angle 45 to 90 to 135.
var Cylon = require('cylon'); Cylon.robot({ connections: { arduino: { adaptor: 'firmata', port: '/dev/ttyACM0' } }, devices: { servo: { driver: 'servo', pin: 3 } }, work: function(my) { var angle = 45 ; my.servo.angle(angle); every((1).second(), function() { angle = angle + 45 ; if (angle > 135) { angle = 45 } my.servo.angle(angle); }); } }).start();
Commands
currentAngle
Returns the current angle of the Servo
Params
-
callback (
Function
) function to be invoked with angle value
Returns
- (
Number
) the current servo angle value (0-180)
angle
Sets the angle of the servo to the provided value
Params
-
value (
Number
) - the angle to point the servo to (0-180) -
[callback] (
Function
) - (err, val) triggers when write is complete
Returns
- (
undefined
)
safeAngle
Given a servo angle, determines if it's safe or not, and returns a safe value
Params
-
value (
Number
) the angle the user wants to set the servo to
Returns
- (
Number
) a made-safe angle to set the servo to
Circuit
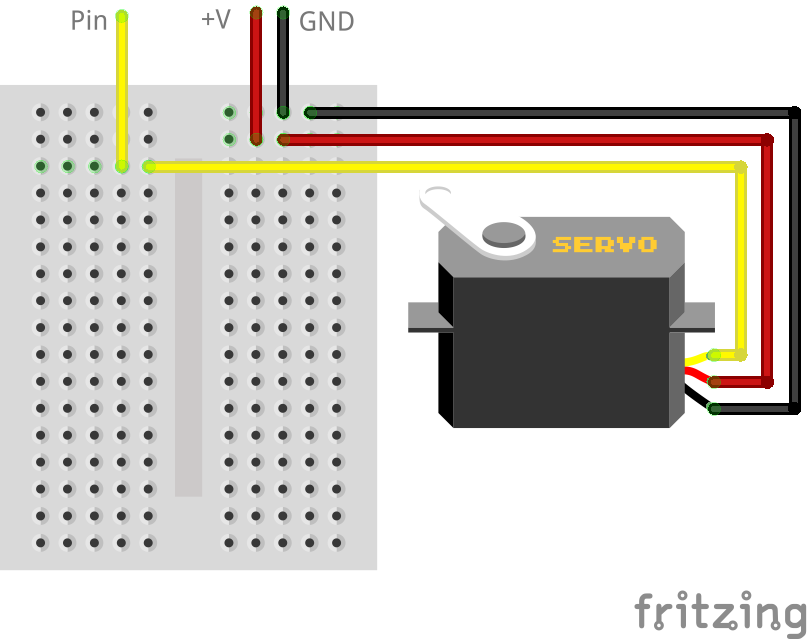