LCD
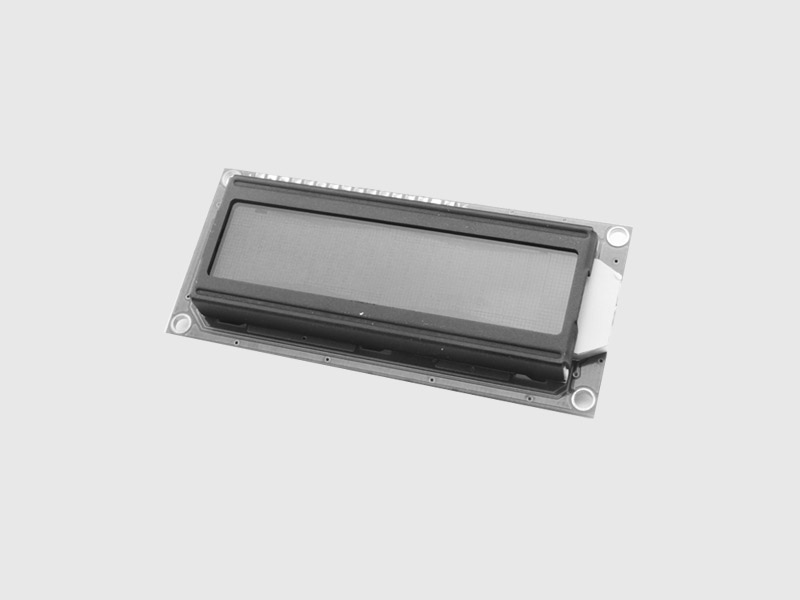
Used for display characters.
For more information click here.
How To Connect
Cylon.robot({ connections: { arduino: { adaptor: 'firmata', port: '/dev/ttyACM0' } }, devices: { lcd: { driver: 'lcd' } }, });
How To Use
Example of the LCD driver with the Arduino.
Cylon = require('cylon'); Cylon.robot({ connections: { arduino: { adaptor: 'firmata', port: '/dev/ttyACM0' } }, devices: { lcd: { driver: 'lcd' } }, work: function(my) { my.lcd.on('start', function(){ my.lcd.print("Hello!"); }); } }).start()
Commands
clear
Clears display and returns cursor to the home position (address 0).
Params
-
[callback] (
Function
) function to be invoked when done
Returns
- (
undefined
)
home
Returns cursor to home position.
Params
-
[callback] (
Function
) function to be invoked when done
Returns
- (
undefined
)
setCursor
Sets cursor position.
Params
-
col (
Number
) cursor column to set -
row (
Number
) cursor row to set -
[callback] (
Function
) function to be invoked when done
Returns
- (
undefined
)
displayOff
Sets Off of all display (D), cursor Off (C) and blink of cursor position character (B).
Params
-
[callback] (
Function
) function to be invoked when done
Returns
- (
undefined
)
displayOn
Sets On of all display (D), cursor On (C) and blink of cursor position character (B).
Params
-
[callback] (
Function
) function to be invoked when done
Returns
- (
undefined
)
cursorOff
Turns off the cursor.
Params
-
[callback] (
Function
) function to be invoked when done
Returns
- (
undefined
)
cursorOn
Turns on the cursor.
Params
-
[callback] (
Function
) function to be invoked when done
Returns
- (
undefined
)
blinkOff
Turns off the cursor blinking character.
Params
-
[callback] (
Function
) function to be invoked when done
Returns
- (
undefined
)
blinkOn
Turns on the cursor blinking character.
Params
-
[callback] (
Function
) function to be invoked when done
Returns
- (
undefined
)
backlightOff
Turns off the back light.
Params
-
[callback] (
Function
) function to be invoked when done
Returns
- (
undefined
)
backlightOn
Turns on the back light.
Params
-
[callback] (
Function
) function to be invoked when done
Returns
- (
undefined
)
Prints characters on the LCD.
Params
-
str (
String
) string to print on the LCD -
[callback] (
Function
) function to be invoked when done
Returns
- (
undefined
)
Circuit
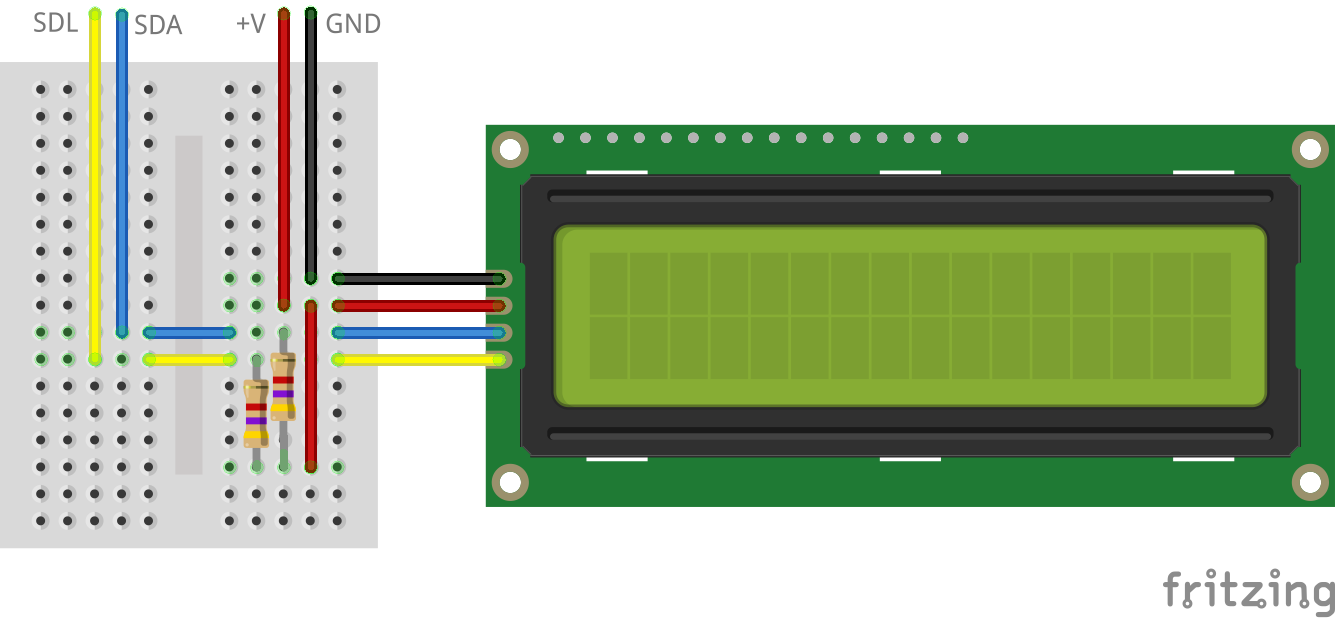