Force
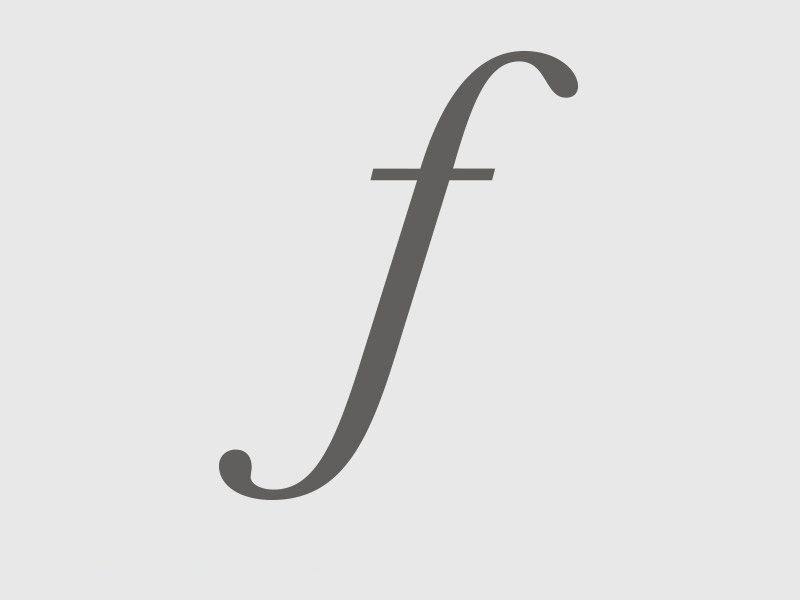
This modules provides an adaptor/driver combination to communicate with Salesforce.
It uses the official JSForce module.
For more info about the SalesForce platform, click here.
How To Connect
Cylon.robot({ connections: { sfcon: { adaptor: 'force', sfuser: process.env.SF_USERNAME, sfpass: process.env.SF_SECURITY_TOKEN } }, devices: { salesforce: { driver: 'force'} },
How To Use
This example pushes data via an Apex rest call, and also subscribes to it using cylon-force
var Cylon = require('cylon'); Cylon.robot({ connections: { sfcon: { adaptor: 'force', sfuser: process.env.SF_USERNAME, sfpass: process.env.SF_SECURITY_TOKEN } }, devices: { salesforce: { driver: 'force'} }, work: function(me) { me.salesforce.subscribe('SpheroMsgOutbound', function(data) { console.log(data); }); var i = 0 ; every((2).seconds(), function() { var toSend = {spheroName: 'globo', bucks: i++} me.salesforce.push('/SpheroController/', toSend); }); } }); Cylon.start();
Commands
subscribe
Subscribes to a topic in Salesforce
Params
-
topic (
String
) name of the topic to subscribe to -
callback (
Function
) triggered when new data is received for the topic
Returns
- (
undefined
)
post
Posts data to a Salesforce Apex Class
Params
-
apexPath (
String
) SF apex path to send data to -
data (
Object
) information to store in Salesforce -
callback (
Function
) triggered when data is sent to SF
Returns
- (
undefined
)
get
Gets data from a Salesforce Apex Class
Params
-
apexPath (
String
) SF apex path to get data from -
callback (
Function
) triggered when new data is received
Returns
- (
undefined
)
query
Queries data from Salesforce
Params
-
query (
String
) SF query to look for data with -
callback (
Function
) triggered when new data is received
Returns
- (
undefined
)