M2X
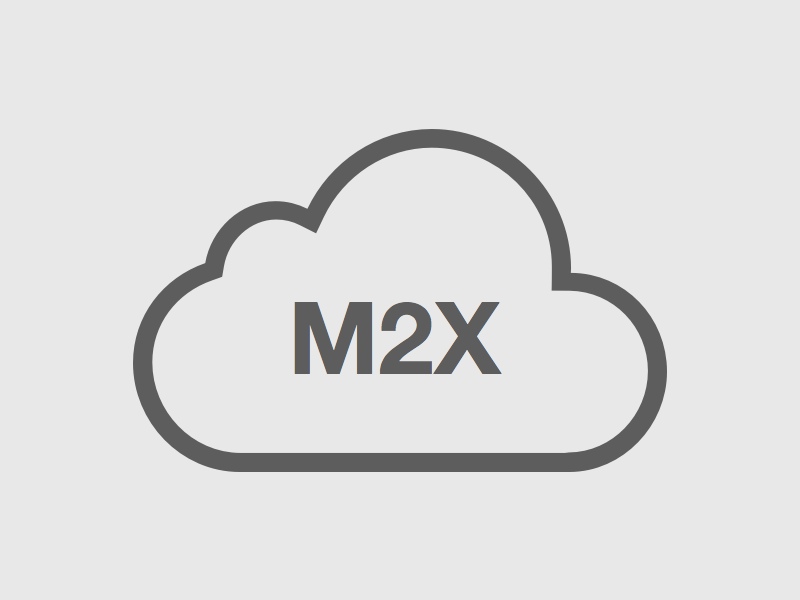
A Cloud-Based Data Storage Service and Management Toolset Customized for the Internet of Things
For more info about the M2X platform click here.
How To Connect
Cylon.robot({ connections: { m2x: { adaptor: 'm2x', apiKey: 'YOUR_API_KEY' feedId: 'YOUR_FEED_ID' } }, devices: { m2x: { driver: 'm2x' } }, });
How To Use
This small program will talk to M2X, incrementing a stream and printing the current value:
var Cylon = require('cylon'); Cylon.robot({ connections: { m2x: { adaptor: 'm2x', apiKey: 'YOUR_API_KEY' feedId: 'YOUR_FEED_ID' } }, devices: { m2x: { driver: 'm2x' } }, work: function(my) { var count = 1; every(2000, function() { my.m2x.push('money', { value: count++ }); }); my.m2x.subscribe('money', function(data) { console.log("Latest value from M2X: " + data); }); } }).start();
Commands
catalog
Retrieves a list of public devices
Params
-
params (
String
) list of params to filter the list of devices -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
list
Retrieves a list of private devices
Params
-
params (
String
) list of params to filter the list of devices -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
groups
Retrieves an array of groups to which the devices belong to
Params
-
params (
String
) list of params to filter for -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
create
Creates a new Device
Params
-
params (
Object
) list of device attrs -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
update
Updates a Device
Params
-
id (
String
) of the device to update -
params (
Object
) list of device attrs -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
view
Returns an object with device details for the specified id
Params
-
id (
String
) of the device -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
location
Returns the location of a device
Params
-
id (
String
) of the device -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
updateLocation
Updates the location of a device
Params
-
id (
String
) of the device -
params (
Object
) to be updated -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
streams
Retrieve a list of streams for the device
Params
-
id (
String
) of the device -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
updateStream
Updates(or creates) stream with the params passed
Params
-
id (
String
) of the device -
name (
String
) of the stream to be updated -
params (
Object
) to be updated -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
setStreamValue
Creates a new stream value
Params
-
id (
String
) of the device -
name (
String
) of the stream to be updated -
params (
Object
) { value: myValue[, timestamp: ISOTimestamp ]} -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
stream
Retrieve stream details
Params
-
id (
String
) of the device -
name (
String
) of the stream to be updated -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
streamValues
Retrieve stream values
Params
-
id (
String
) of the device -
name (
String
) of the stream to be updated -
filters (
Object
) for the values -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
sampleStreamValues
Retrieve a sample of stream values
Params
-
id (
String
) of the device -
name (
String
) of the stream to be updated -
filters (
Object
) for the values -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
streamStats
Retrieve stream stats
Params
-
id (
String
) of the device -
name (
String
) of the stream to be updated -
filters (
Object
) for the values -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
postValues
Add multiple values to a stream
Params
-
id (
String
) of the device -
name (
String
) of the stream to be updated -
values (
array
) array of { value: val, timestamp: ISODateString } -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
deleteStreamValues
Deletes stream values
Params
-
id (
String
) of the device -
name (
String
) of the stream to be updated -
filters (
Object
) to delete values -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
deleteStream
Deletes a stream
Params
-
id (
String
) of the device -
name (
String
) of the stream to be updated -
filters (
String
) filters for deleted stream -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
postMultiple
Post to multiple streams
Params
-
id (
String
) of the device -
values (
Array
) array of { name: n, value: v, timestamp: t } -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
triggers
returns a list of triggers for the device
Params
-
id (
String
) of the device -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
createTrigger
creates a new trigger
Params
-
id (
String
) of the device -
params (
Object
) for the trigger -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
trigger
creates a new trigger
Params
-
id (
String
) of the device -
triggerId (
String
) of the trigger -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
updateTrigger
updates a trigger
Params
-
id (
String
) of the device -
name (
String
) of the trigger -
params (
Object
) to be updated -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
testTrigger
tests a trigger
Params
-
id (
String
) of the device -
name (
String
) of the trigger -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
deleteTrigger
deletes a trigger
Params
-
id (
String
) of the device -
name (
String
) of the trigger -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
log
Returns a log of access to the supplied device
Params
-
id (
String
) of the device -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
deleteDevice
deletes a device
Params
-
id (
String
) of the device -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
keys
returns a list of keys associated to a device
Params
-
id (
String
) of the device -
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
createKey
creates a new key
Params
-
id (
String
) of the device -
params (obejct) to create the new key
-
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)
updateKey
updates a key
Params
-
id (
String
) of the device -
key (
String
) key to update -
params (obejct) to update the key
-
callback (standardCallback) - Triggers with (err, data)
Returns
- (
undefined
)