MiP
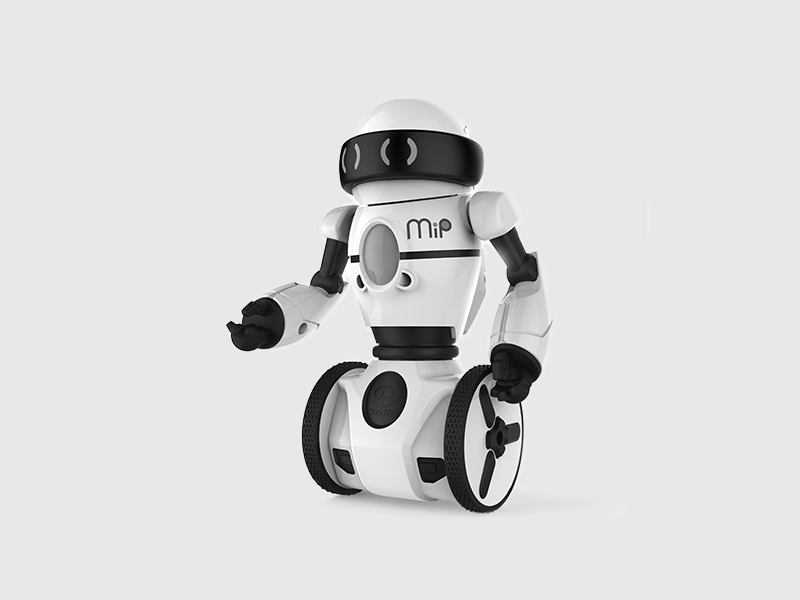
This is the Cylon driver for the MiP allowing you to interract with its many capabilities.
How To Connect
You need to determine the uuid
of your MiP. One way to do this, is to use the cylon-ble-scan
command line utility installed as part of cylon-ble.
Once you know your uuid
just substititute it into your code.
How To Use
var Cylon = require('cylon'); Cylon.robot({ connection: { name: 'bluetooth', adaptor: 'central', uuid: 'd03972a24e55', module: 'cylon-ble'}, devices: [{name: 'mip', driver: 'mip'}], work: function(my) { my.mip.setHeadLED(2, 2, 2, 2); after((2).seconds(), function() { my.mip.distanceDrive(0, 10, 0, 0); }); after((3).seconds(), function() { my.mip.setHeadLED(1, 1, 1, 1); }); } }).start();
Commands
setHeadLED
Sets the Head LEDs of the MiP
Params
-
light1 (
Number
) light one value -
light2 (
Number
) light two value -
light3 (
Number
) light three value -
light4 (
Number
) light four value -
callback (
Function
) function to call when done
Returns
- (
undefined
)
setChestLED
Sets the Chest LED color of the MiP
Params
-
r (
Number
) red value -
g (
Number
) green value -
b (
Number
) blue value -
callback (
Function
) function to call when done
Returns
- (
undefined
)
flashChestLED
Flashes the chest LED of the MiP
Params
-
r (
Number
) red value -
g (
Number
) green value -
b (
Number
) blue value -
timeOn (
Number
) time on -
timeOff (
Number
) time off -
callback (
Function
) function to call when done
Returns
- (
undefined
)
getUp
Tells the MiP to get up.
Params
-
stand (
Number
) MiP should stand -
callback (
Function
) function to call when done
Returns
- (
undefined
)
driveDistance
Tells the MiP to drive a distance, in a direction
Params
-
direction (
Number
) direction to drive -
distance (
Number
) distance to drive -
turnDirection (
Number
) direction to turn -
turnAngle (
Number
) angle to turn -
callback (
Function
) function to call when done
Returns
- (
undefined
)
driveForward
Tells the MiP to drive forward
Params
-
speed (
Number
) speed to move at -
time (
Number
) how long to move -
callback (
Function
) function to call when done
Returns
- (
undefined
)
driveBackward
Tells the MiP to drive backward
Params
-
speed (
Number
) speed to move at -
time (
Number
) how long to move -
callback (
Function
) function to call when done
Returns
- (
undefined
)
turnLeft
Tells the MiP to turn to the left
Params
-
angle (
Number
) angle to turn to -
speed (
Number
) speed to turn at -
callback (
Function
) function to call when done
Returns
- (
undefined
)
turnRight
Tells the MiP to turn to the right
Params
-
angle (
Number
) angle to turn to -
speed (
Number
) speed to turn at -
callback (
Function
) function to call when done
Returns
- (
undefined
)
setGameMode
Tells the MiP to start the specified game mode
Params
-
mode (
Number
) mode to set to -
callback (
Function
) function to call when done
Returns
- (
undefined
)
stop
Tells the MiP to stop whatever it's doing.
Params
-
callback (
Function
) function to call when done
Returns
- (
undefined
)