Tessel Infrared
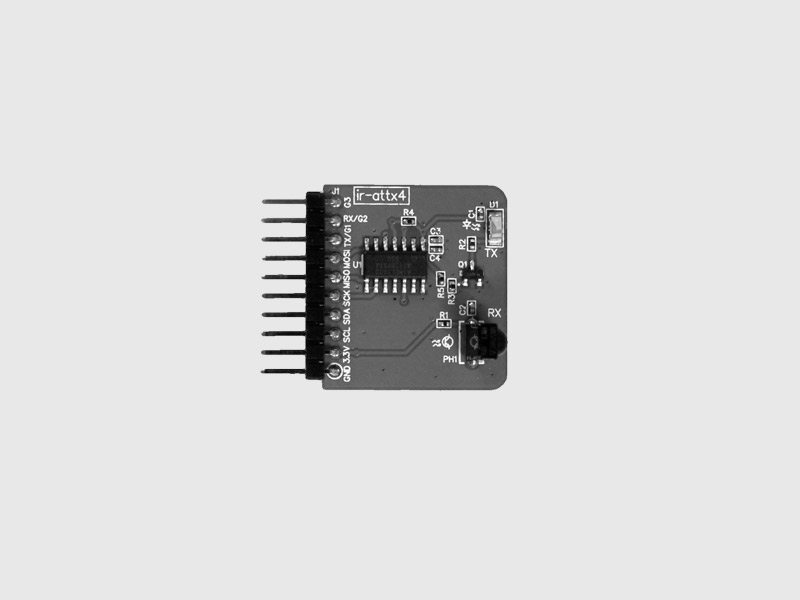
The Infrared (IR) Module can send and detect IR signals.
Use a Tessel as a remote for your TV, radio, or even another Tessel.
- Transmits and receives from 30+ feet (line of sight)
- Detects max 38kHz
- IR Receiver
- IR LED
For more info visit here.
How To Connect
Cylon.robot({ connections: { tessel: { adaptor: 'tessel', port: 'A' } }, devices: { ir: { driver: 'ir-attx4' } }, });
How To Use
Example using a Direct Pin.
var Cylon = require('cylon'); Cylon.robot({ connections: { tessel: { adaptor: 'tessel', port: 'A' } }, devices: { ir: { driver: 'ir-attx4' } }, work: function(my) { my.ir.on('error', function (err) { console.log(err) }); my.ir.on('data', function(data) { console.log("Received RX Data: ", data); }); every((3).seconds(), function() { /********************************************* This infrared module example transmits the power signal sequence of an Insignia brand television every three seconds, while also listening for (and logging) any incoming infrared data. *********************************************/ // Make a buffer of on/off durations (each duration is 16 bits) var powerBuffer = new Buffer([0, 178, 255, 168, 0, 12, 255, 246, 0, 13, 255, 225, 0, 13, 255, 224, 0, 12, 255, 246, 0, 12, 255, 246, 0, 13, 255, 247, 0, 13, 255, 247, 0, 13, 255, 224, 0, 12, 255, 224, 0, 13, 255, 247, 0, 13, 255, 224, 0, 12, 255, 246, 0, 12, 255, 246, 0, 12, 255, 246, 0, 12, 255, 246, 0, 13, 255, 247, 0, 13, 255, 224, 0, 12, 255, 224, 0, 13, 255, 225, 0, 13, 255, 224, 0, 12, 255, 246, 0, 12, 255, 246, 0, 13, 255, 247, 0, 13, 255, 247, 0, 13, 255, 246, 0, 12, 255, 246, 0, 12, 255, 246, 0, 12, 255, 246, 0, 12, 255, 224, 0, 13, 255, 224, 0, 12, 255, 224, 0, 12, 255, 224, 0, 12]); // Send the signal at 38 kHz my.ir.sendRawSignal(38, powerBuffer, function(err) { if (err) { console.log("Unable to send signal: ", err); } else { console.log("Signal sent!"); } }); }); } }).start();
Commands
infrared.sendRawSignal(frequency, signalDurations, callback)
The primary method for sending data. The first argument is a frequency of signal in Hz, typically 38 but can range from 36 to 40. The second argument is a buffer of unsigned 16 bit integers representing the number of microseconds the transmission should be on. The max length of the signal durations is 100 durations.
infrared.setListening(set, callback)
Determines whether the module is listening for incoming signals. Will automatically be set and unset depending on listeners for the data event.
Events
'data'
Emitted when an infrared signal is detected.
'error'
Emitted when there is an error communicating with the module.
'ready'
Emitted upon first successful communication between the Tessel and the module.